Understanding TypeScript
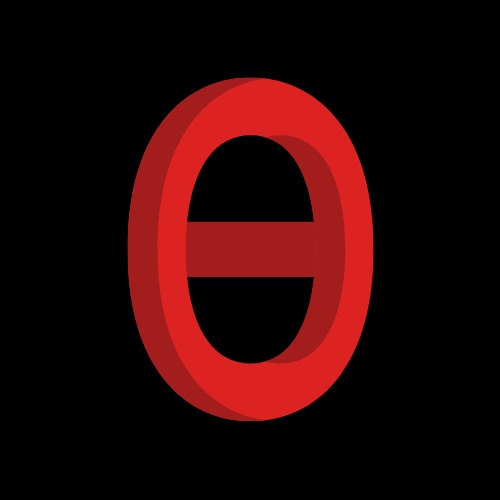
About Course
Learn TypeScript for free with this comprehensive course from Udemy. This course takes you from the very basics to advanced concepts like decorators and workflows with TypeScript and webpack.
This course is designed for both beginners and experienced JavaScript developers who want to learn TypeScript. It covers all the essential concepts of TypeScript, including:
* Types and how to use them
* How the TypeScript compiler works
* ES6 features with TypeScript
* Classes in TypeScript
* Namespaces and modules
* Interfaces
* Generics
* Decorators
* Integrating third-party JavaScript libraries into your TypeScript projects
* Setting up a TypeScript project with webpack
* Using TypeScript with ReactJS
* Using TypeScript with Node/Express
* Real projects and use-cases
This course includes high-quality learning videos and plenty of exercises with solutions to help you practice what you learn. Join now and gain an edge in the world of web development with TypeScript.
What Will You Learn?
- Use TypeScript and its Features like Types, ES6 Support, Classes, Modules, Interfaces and much more in any of their Projects
- Understand what TypeScript really is about and how it works
- Why TypeScript offers a real advantage over vanilla JavaScript
- Learn TypeScript both in theory as well as applied to real use-cases and projects
- Learn how to combine TypeScript with ReactJS or NodeJS / Express
Course Content
1 – Getting Started
-
A Message from the Professor
-
002 – What Is TypeScript Why Should You Use It.mp4
00:00 -
003 – Join Our Learning Community.html
00:00 -
004 – Installing Using TypeScript.mp4
00:00 -
005 – TypeScript Advantages Overview.mp4
00:00 -
006 – Course Outline.mp4
00:00 -
007 – How To Get The Most Out Of The Course.mp4
00:00 -
008 – Setting Up A Code Editor IDE.mp4
00:00 -
009 – The Course Project Setup.mp4
00:00
2 – TypeScript Basics Basic Types
-
010 – Module Introduction.mp4
00:00 -
011 – Using Types.mp4
00:00 -
012 – TypeScript Types vs JavaScript Types.mp4
00:00 -
013 – Important Type Casing.html
00:00 -
014 – Working with Numbers Strings Booleans.mp4
00:00 -
015 – Type Assignment Type Inference.mp4
00:00 -
016 – Object Types.mp4
00:00 -
017 – Nested Objects Types.html
00:00 -
018 – Arrays Types.mp4
00:00 -
019 – Working with Tuples.mp4
00:00 -
020 – Working with Enums.mp4
00:00 -
021 – The any Type.mp4
00:00 -
022 – Union Types.mp4
00:00 -
023 – Literal Types.mp4
00:00 -
024 – Type Aliases Custom Types.mp4
00:00 -
025 – Type Aliases Object Types.html
00:00 -
026 – Function Return Types void.mp4
00:00 -
027 – Functions as Types.mp4
00:00 -
028 – Function Types Callbacks.mp4
00:00 -
029 – The unknown Type.mp4
00:00 -
030 – The never Type.mp4
00:00 -
031 – Wrap Up.mp4
00:00 -
032 – Useful Resources Links.html
00:00 -
Section Quiz
3 – The TypeScript Compiler and its Configuration
-
033 – Module Introduction.mp4
00:00 -
034 – Using Watch Mode.mp4
00:00 -
035 – Compiling the Entire Project Multiple Files.mp4
00:00 -
036 – Including Excluding Files.mp4
00:00 -
037 – Setting a Compilation Target.mp4
00:00 -
038 – Understanding TypeScript Core Libs.mp4
00:00 -
039 – More Configuration Compilation Options.mp4
00:00 -
040 – Working with Source Maps.mp4
00:00 -
041 – rootDir and outDir.mp4
00:00 -
042 – Stop Emitting Files on Compilation Errors.mp4
00:00 -
043 – Strict Compilation.mp4
00:00 -
044 – Code Quality Options.mp4
00:00 -
045 – Debugging with Visual Studio Code.mp4
00:00 -
046 – Wrap Up.mp4
00:00 -
047 – Useful Resources Links.html
00:00 -
Section Quiz
4 – Nextgeneration JavaScript TypeScript
-
048 – Module Introduction.mp4
00:00 -
049 – let and const.mp4
00:00 -
050 – Arrow Functions.mp4
00:00 -
051 – Default Function Parameters.mp4
00:00 -
052 – The Spread Operator.mp4
00:00 -
053 – Rest Parameters.mp4
00:00 -
054 – Array Object Destructuring.mp4
00:00 -
055 – How Code Gets Compiled Wrap Up.mp4
00:00 -
056 – Useful Resources Links.html
00:00 -
Section Quiz
5 – Classes Interfaces
-
057 – Module Introduction.mp4
00:00 -
058 – What are Classes.mp4
00:00 -
059 – Creating a First Class.mp4
00:00 -
060 – Compiling to JavaScript.mp4
00:00 -
061 – Constructor Functions The this Keyword.mp4
00:00 -
062 – private and public Access Modifiers.mp4
00:00 -
063 – Shorthand Initialization.mp4
00:00 -
064 – readonly Properties.mp4
00:00 -
065 – Inheritance.mp4
00:00 -
066 – Overriding Properties The protected Modifier.mp4
00:00 -
067 – Getters Setters.mp4
00:00 -
068 – Static Methods Properties.mp4
00:00 -
069 – Abstract Classes.mp4
00:00 -
070 – Singletons Private Constructors.mp4
00:00 -
071 – Classes A Summary.mp4
00:00 -
072 – A First Interface.mp4
00:00 -
073 – Using Interfaces with Classes.mp4
00:00 -
074 – Why Interfaces.mp4
00:00 -
075 – Readonly Interface Properties.mp4
00:00 -
076 – Extending Interfaces.mp4
00:00 -
077 – Interfaces as Function Types.mp4
00:00 -
078 – Optional Parameters Properties.mp4
00:00 -
079 – Compiling Interfaces to JavaScript.mp4
00:00 -
080 – Wrap Up.mp4
00:00 -
081 – Useful Resources Links.html
00:00 -
Section Quiz
6 – Advanced Types
-
082 – Module Introduction.mp4
00:00 -
083 – Intersection Types.mp4
00:00 -
084 – More on Type Guards.mp4
00:00 -
085 – Discriminated Unions.mp4
00:00 -
086 – Type Casting.mp4
00:00 -
087 – Index Properties.mp4
00:00 -
088 – Function Overloads.mp4
00:00 -
089 – Optional Chaining.mp4
00:00 -
090 – Nullish Coalescing.mp4
00:00 -
091 – Wrap Up.mp4
00:00 -
092 – Useful Resources Links.html
00:00 -
Section Quiz
7 – Generics
-
093 – Module Introduction.mp4
00:00 -
094 – Builtin Generics What are Generics.mp4
00:00 -
095 – Creating a Generic Function.mp4
00:00 -
096 – Working with Constraints.mp4
00:00 -
097 – Another Generic Function.mp4
00:00 -
098 – The keyof Constraint.mp4
00:00 -
099 – Generic Classes.mp4
00:00 -
100 – A First Summary.mp4
00:00 -
101 – Generic Utility Types.mp4
00:00 -
102 – Generic Types vs Union Types.mp4
00:00 -
103 – Useful Resources Links.html
00:00 -
Section Quiz
8 – Decorators
-
104 – Module Introduction.mp4
00:00 -
105 – A First Class Decorator.mp4
00:00 -
106 – Working with Decorator Factories.mp4
00:00 -
107 – Building More Useful Decorators.mp4
00:00 -
108 – Adding Multiple Decorators.mp4
00:00 -
109 – Diving into Property Decorators.mp4
00:00 -
110 – Accessor Parameter Decorators.mp4
00:00 -
111 – When Do Decorators Execute.mp4
00:00 -
112 – Returning and changing a Class in a Class Decorator.mp4
00:00 -
113 – Other Decorator Return Types.mp4
00:00 -
114 – Example Creating an Autobind Decorator.mp4
00:00 -
115 – Validation with Decorators First Steps.mp4
00:00 -
116 – Validation with Decorators Finished.mp4
00:00 -
117 – Fixing a Validator Bug.html
00:00 -
118 – Wrap Up.mp4
00:00 -
119 – Useful Resources Links.html
00:00 -
Section Quiz
9 – Practice Time Lets build a Drag Drop Project
-
120 – Module Introduction.mp4
00:00 -
121 – Getting Started.mp4
00:00 -
122 – DOM Element Selection OOP Rendering.mp4
00:00 -
123 – Interacting with DOM Elements.mp4
00:00 -
124 – Creating Using an Autobind Decorator.mp4
00:00 -
125 – Fetching User Input.mp4
00:00 -
126 – Creating a ReUsable Validation Functionality.mp4
00:00 -
127 – Rendering Project Lists.mp4
00:00 -
128 – Managing Application State with Singletons.mp4
00:00 -
129 – More Classes Custom Types.mp4
00:00 -
130 – Filtering Projects with Enums.mp4
00:00 -
131 – Adding Inheritance Generics.mp4
00:00 -
132 – Rendering Project Items with a Class.mp4
00:00 -
133 – Using a Getter.mp4
00:00 -
134 – Utilizing Interfaces to Implement Drag Drop.mp4
00:00 -
135 – Drag Events Reflecting the Current State in the UI.mp4
00:00 -
136 – Adding a Droppable Area.mp4
00:00 -
137 – Finishing Drag Drop.mp4
00:00 -
138 – Wrap Up.mp4
00:00 -
139 – Useful Resources Links.html
00:00 -
Section Quiz
10 – Modules Namespaces
-
140 – Module Introduction.mp4
00:00 -
141 – Writing Module Code Your Options.mp4
00:00 -
142 – Working with Namespaces.mp4
00:00 -
143 – Organizing Files Folders.mp4
00:00 -
144 – A Problem with Namespace Imports.mp4
00:00 -
145 – Important Use Chrome or Firefox.html
00:00 -
146 – Using ES Modules.mp4
00:00 -
147 – Understanding various Import Export Syntaxes.mp4
00:00 -
148 – How Does Code In Modules Execute.mp4
00:00 -
149 – Wrap Up.mp4
00:00 -
150 – Useful Resources Links.html
00:00 -
Section Quiz
11 – Using Webpack with TypeScript
-
151 – Module Introduction.mp4
00:00 -
152 – What is Webpack Why do we need it.mp4
00:00 -
153 – Installing Webpack Important Dependencies.mp4
00:00 -
154 – Adding Entry Output Configuration.mp4
00:00 -
155 – Adding TypeScript Support with the tsloader Package.mp4
00:00 -
156 – Adjust Webpack Config.html
00:00 -
157 – Finishing the Setup Adding webpackdevserver.mp4
00:00 -
158 – Adding a Production Workflow.mp4
00:00 -
159 – Wrap Up.mp4
00:00 -
160 – Useful Resources Links.html
00:00
12 – 3rd Party Libraries TypeScript
-
161 – Module Introduction.mp4
00:00 -
162 – Using JavaScript Libraries with TypeScript.mp4
00:00 -
163 – Using declare as a Last Resort.mp4
00:00 -
164 – No Types Needed classtransformer.mp4
00:00 -
165 – TypeScriptembracing classvalidator.mp4
00:00 -
166 – Wrap Up.mp4
00:00 -
167 – Useful Resources Links.html
00:00 -
Section Quiz
13 – Time to Practice Lets build a Select Share a Place App incl Google Maps
-
168 – Module Introduction.mp4
00:00 -
169 – Project Setup.mp4
00:00 -
170 – Getting User Input.mp4
00:00 -
171 – Setting Up a Google API Key.mp4
00:00 -
172 – Using Axios to Fetch Coordinates for an Entered Address.mp4
00:00 -
173 – Rendering a Map with Google Maps incl Types.mp4
00:00 -
174 – Working with Maps without a Credit Card.html
00:00 -
175 – Useful Resources Links.html
00:00 -
Section Quiz
14 – Reactjs TypeScript
-
176 – Module Introduction.mp4
00:00 -
177 – Setting Up a React TypeScript Project.mp4
00:00 -
178 – How Do React TypeScript Work Together.mp4
00:00 -
179 – Working with Props and Types for Props.mp4
00:00 -
180 – Getting User Input with refs.mp4
00:00 -
181 – CrossComponent Communication.mp4
00:00 -
182 – Working with State Types.mp4
00:00 -
183 – Managing State Better.mp4
00:00 -
184 – More Props State Work.mp4
00:00 -
185 – Adding Styling.mp4
00:00 -
186 – Types for other React Features eg Redux or Routing.mp4
00:00 -
187 – Wrap Up.mp4
00:00 -
188 – Useful Resources Links.html
00:00 -
Section Quiz
15 – Nodejs Express TypeScript
-
189 – Module Introduction.mp4
00:00 -
190 – Executing TypeScript Code with Nodejs.mp4
00:00 -
191 – Setting up a Project.mp4
00:00 -
192 – Finished Setup Working with Types in Node Express Apps.mp4
00:00 -
193 – Adding Middleware Types.mp4
00:00 -
194 – Working with Controllers Parsing Request Bodies.mp4
00:00 -
195 – More CRUD Operations.mp4
00:00 -
196 – Wrap Up.mp4
00:00 -
197 – Useful Resources Links.html
00:00 -
Section Quiz
16 – Course Roundup
-
198 – Thanks for being part of the course.mp4
00:00
Earn a certificate
Add this certificate to your resume to demonstrate your skills & increase your chances of getting noticed.
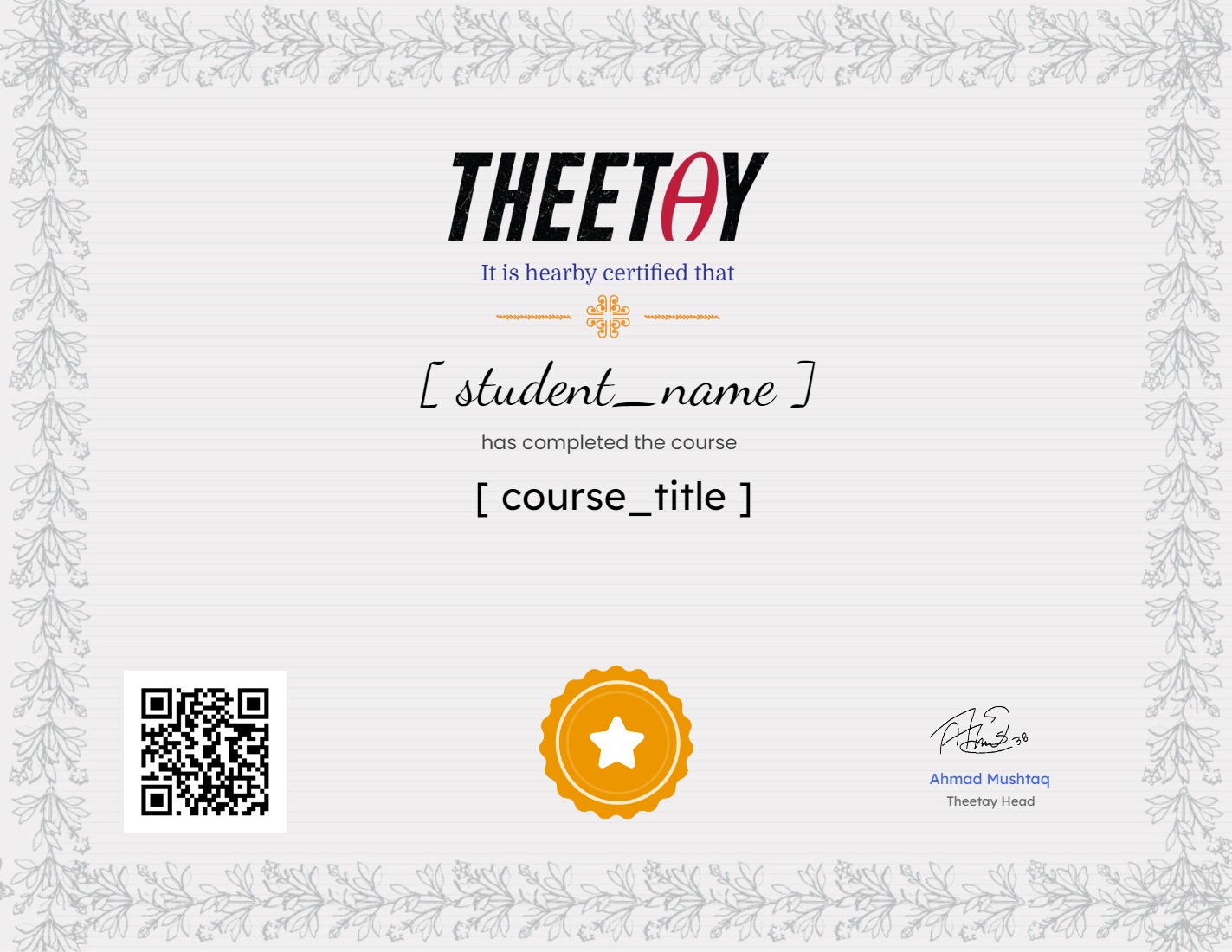