The Complete Spring Boot Development Bootcamp
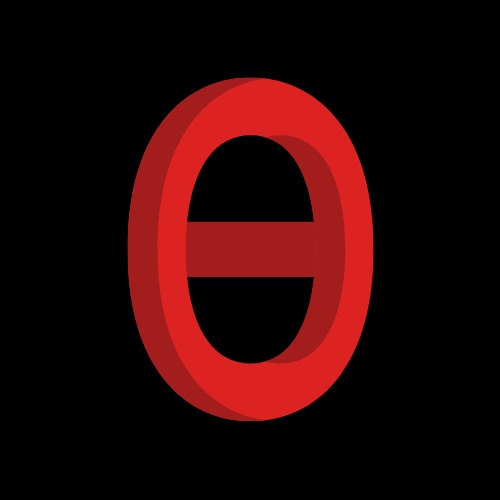
About Course
Learn how to build powerful web applications with Spring Boot, the best Java framework for web development. This free course from Udemy will teach you everything you need to know to become a Java Web Developer, including:
- Setting up a Spring Boot Project
- Model View Controller (MVC)
- Field Validation
- Three-Layer Codebase
- Bean and Dependency Injection
- Testing a Spring Boot Web Application
- React Integration
- REST API Development
- OpenAPI Documentation
- Exception Handling
- Spring Data JPA (SQL) with Relationships
- Spring Boot Security (Basic and JWT Authentication)
This interactive course is packed with coding exercises to help you learn by doing. It’s a great way to get started with Spring Boot and build your skills as a Java Web Developer. This course is completely free and is offered by Udemy, one of the leading online learning platforms. Take this course today and start building your dream web applications!
What Will You Learn?
- Launch an HTTP Server
- Model View Controller
- Field Validation
- Beans and Dependency Injection
- Unit Testing Business Logic.
- Integration Testing the Request – Response Lifecycle.
- REST API
- Exception Handling
- OpenAPI Documentation
- Spring Data JPA (SQL Database)
- Spring Security (Basic)
- Spring Security (JWT)
Course Content
Module 1: Spring Boot Fundamentals
-
A Message from the Professor
-
– Introduction
00:25 -
– Prerequisite for this course
00:21 -
– Where to Ask Questions
00:30
Required Installations
-
– Required Installation Java 17 Mac
02:05 -
– Required Installation Java 17 Windows
01:55 -
– Recommended Installation Maven Mac
04:40 -
– Recommended Installation Maven Windows
04:30
Choose Your Path
-
– Visual Studio Code Vs IntellJ
01:58
PATH A: Visual Studio Code (Recommended)
-
– Roadmap
00:16 -
– Downloading Visual Studio Code
01:43 -
– Creating a Spring Boot Project
04:39 -
– Breaking Down a Spring Boot Project
02:27 -
– Running a Spring Boot Application
04:46 -
– The ClientServer Model
05:50 -
– Workbook and Challenges
01:39 -
– Workbook 11
00:42 -
– Launching an HTTP Server
09:42 -
– Workbook 12
01:21 -
– Workbook 13
01:04 -
– Maven Dependencies
04:17 -
– Spring Boot DevTools Dependency
03:13 -
– Cheat Sheet
00:15
Challenge 1
-
– Challenge
00:38 -
– Solution
07:13
Part B: IntelliJ
-
– Roadmap
00:16 -
– Downloading IntelliJ
02:24 -
– Creating a Spring Boot Project
04:02 -
– Breaking Down a Spring Boot Project
02:27 -
– Running a Spring Boot Application
05:02 -
– The ClientServer Model
05:50 -
– Workbooks and Challenges
01:39 -
– Workbook 11
00:42 -
– Launching an HTTP Server
09:59 -
– Workbook 12
01:21 -
– Workbook 13
01:04 -
– Maven Dependencies
04:17 -
– Spring Boot DevTools Dependency
03:04 -
– Cheat Sheet
00:15
Challenge 1
-
– Challenge
00:38 -
– Solution
08:03
Model View Controller
-
– Roadmap
01:00 -
– Roadmap
01:00 -
– Starter Project
01:14 -
– The MVC Design
02:38 -
– The Controller
05:16 -
– Path A Breakpoint Session
02:07 -
– Path B Breakpoint Session
02:07 -
– The View
02:43 -
– Backend vs Front end
00:44 -
– Path A The Model
06:39 -
– Path B The Model
06:40 -
– Path A Breakpoint Session
01:15 -
– Path B Breakpoint Session
01:25 -
– Thymeleaf Combining Model and View
05:55 -
– Selection Expression
03:09 -
– Thymeleaf Conditionals
09:00 -
– Utility Methods
07:23 -
– Link Expression
05:48 -
– Thymeleaf Loops
08:52 -
– Path A Breakpoint Session
01:43 -
– Path B Breakpoint Session
01:53 -
– Creating a Form
05:52 -
– Form Submission
09:16 -
– Path A Breakpoint Session
05:21 -
– Path B Breakpoint Session
05:21 -
– Updating Student Grade
14:51 -
– Path A Breakpoint Session
06:06 -
– Path B Breakpoint Session
06:16 -
– Updating Grade Based on Id
07:27 -
– Path A Breakpoint Session
09:52 -
– Path B Breakpoint Session
10:00 -
– Final Touches
03:26 -
– Final Touches
03:26
Challenge 2
-
– Challenge
01:11 -
– Solution Part 1
10:09 -
– Solution Part 2
15:25 -
– Solution Part 2 Followup
12:38 -
– Solution Part 3 Tasks 1 4
14:05 -
– Solution Part 3 Remaining Tasks
12:21
Field Validation
-
– Roadmap
00:21 -
– Field Validation
11:46 -
– Breakpoint Session
03:50 -
– Breakpoint Session
03:50 -
– Workbook 31
00:37 -
– Custom Constraints
10:52 -
– Workbook 32 incl Cross Field Validation
00:30 -
– Workbook 33
00:26 -
– Breakpoint Session
03:05 -
– Breakpoint Session
03:15
Challenge 3
-
– Challenge
00:44 -
– Solution
14:00
Three Layer Codebase
-
– Three Layer Architecture
04:16 -
– Repository
08:20 -
– Service
09:05
Challenge 4
-
– Challenge
00:17 -
– Solution
12:23
Beans and Dependency Injection
-
– Beans
01:57 -
– Dependency Injection
05:08 -
– Service and Repository
02:51 -
– Bean
03:45 -
– Workbook 51
00:44 -
– Breakpoint Session
05:20
Challenge 5
-
– Autowired Vs Constructor
01:36 -
– Solution
05:40
Testing a Web Application
-
– The Importance of Dependency Injection for Unit Testing
03:52 -
– Setting up Testing Class
05:33 -
– Unit Testing the Service Class
19:06 -
– Breakpoint Session
06:09 -
– Intro to Integration Testing
04:10 -
– Integration Testing Part 1
04:07 -
– Integration Testing Part 2
12:56 -
– Breakpoint Session
04:40
React
-
– React Frontend and Spring Boot Backend
05:01 -
– Demo React Spring Boot
04:35
Module 2: Backend Development
-
– Module 2
00:37
REST API
-
– REST API
03:47 -
– REST API Getting Started
06:59 -
– REST API GET Operation
13:24 -
– Postman
01:35 -
– REST API POST Operation
08:40 -
– REST API PUT Operation
06:02 -
– REST API DELETE Operation
08:47
Challenge 6: OpenAPI Documentation
-
– Solution
20:06
Challenge 7: Testing
-
– Solution
19:27
SQL Database (Spring Boot JPA)
-
– Roadmap
00:42 -
– Getting Started JPA and H2
05:21 -
– Object Relational Mapper
08:17 -
– Saving a Student
11:41 -
– Retrieving a Student
03:17 -
– Deleting a Student
03:16 -
– Lombok
03:39 -
– Grade Entity
05:14 -
– Unidirectional Many to One
09:21 -
– Read Grade from Student ID
04:06 -
– Bidirectional One to Many
11:18 -
– Cascade
02:12 -
– Autowired vs AllArgsConstructor
02:31 -
– Course Entity
09:56 -
– Bidirectional One to Many
10:08 -
– Refactoring Around Optionals
07:31 -
– Finalizing the GradeServiceImpl
07:27 -
– Constraints Preventing Duplicate Grades
02:54 -
– Refactoring Around Optionals Loose Ends
05:13
Challenge 8: Many to Many Relationship
-
– Solution
23:22 -
– Solution
04:17
Spring Security: Basic and JWT Authentication (featuring MySQL)
-
– Roadmap
01:09 -
– Basic Auth Authentication and Authorization
03:52 -
– Basic Auth with Spring Security Part 1
18:34 -
– Basic Auth with Spring Security Part 2
08:53 -
– Getting Started JWT Project
06:51 -
– Tokenbased Authentication JWT
10:07 -
– Tokenbased Authentication Part 1
06:17 -
– Intermission Protecting the Password
01:28 -
– Tokenbased Authentication Part 2
09:49 -
– Side Quest Exception Handling Dispatcher Servlet
13:43 -
– Tokenbased Authentication Part 3
16:59 -
– Tokenbased Authentication Part 4
10:11 -
– Tokenbased Authentication Part 5
29:06 -
– Final Recap
03:43
Appendix A: Helpful Resources
-
– Path A Customize your Editor
02:47 -
– Path B Customize your Editor
01:48 -
– Path A Breakpoints in Visual Studio Code
05:30 -
– Path B Breakpoints in IntelliJ
05:17
Appendix B: Workbook Solutions
-
– Workbook 11
01:16 -
– Path A Workbook 12
04:40 -
– Path B Workbook 12
04:07 -
– Path A Workbook 13
03:57 -
– Path B Workbook 13
04:02 -
– Workbook 21
05:41 -
– Workbook 22
09:03 -
– Workbook 23
03:50 -
– Workbook 24
02:08 -
– Workbook 25
05:42 -
– Workbook 26
05:06 -
– Workbook 27
04:49 -
– Workbook 210
04:29 -
– Workbook 211
09:23 -
– Workbook 31
22:01 -
– Workbook 32
03:41 -
– Workbook 33
12:59 -
– Workbook 51
04:39 -
– Workbook 81
09:16 -
– Workbook 82
11:17 -
– Workbook 83
09:18 -
– Workbook 91
10:36 -
– Workbook 101
07:21
Earn a certificate
Add this certificate to your resume to demonstrate your skills & increase your chances of getting noticed.
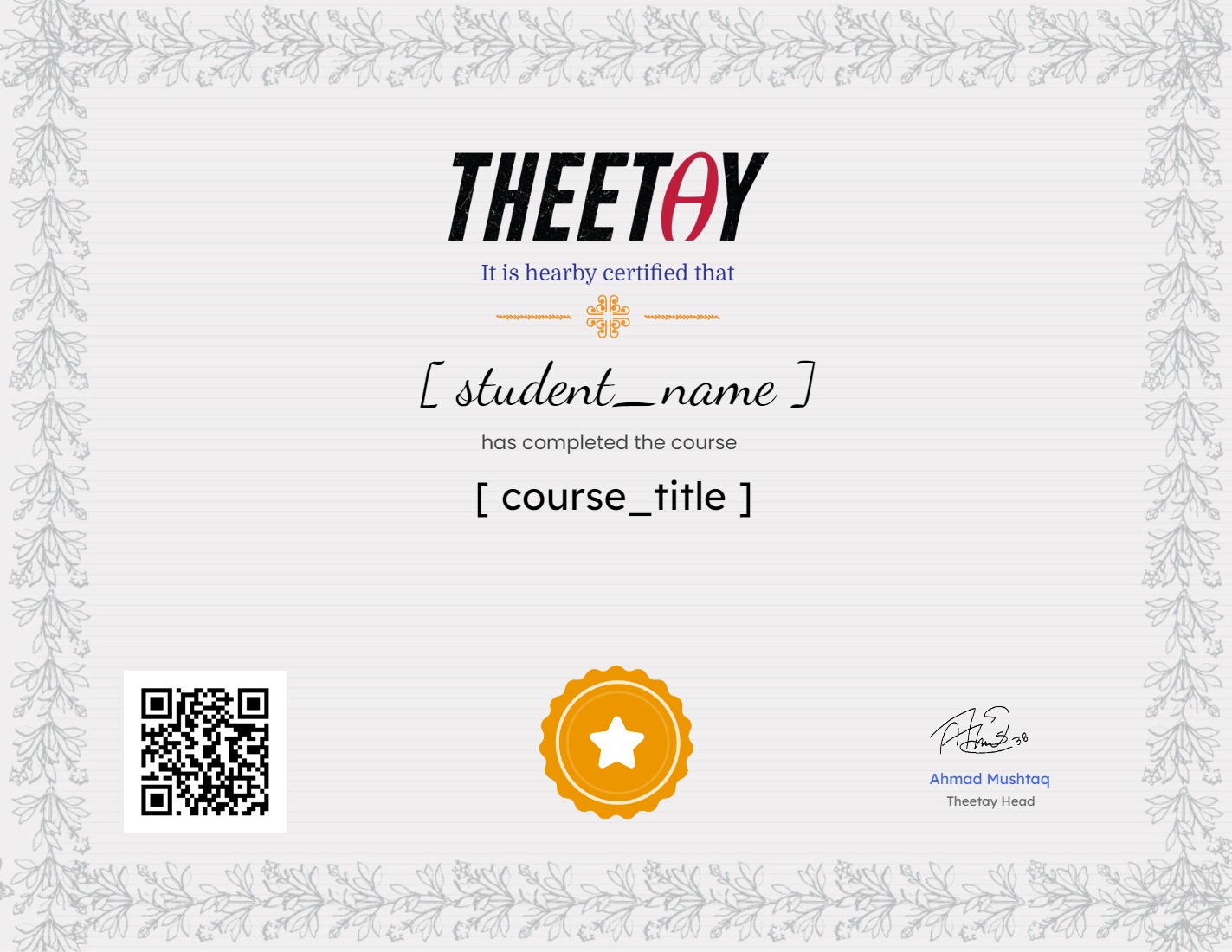
Student Ratings & Reviews
No Review Yet