The Advanced Web Developer Bootcamp
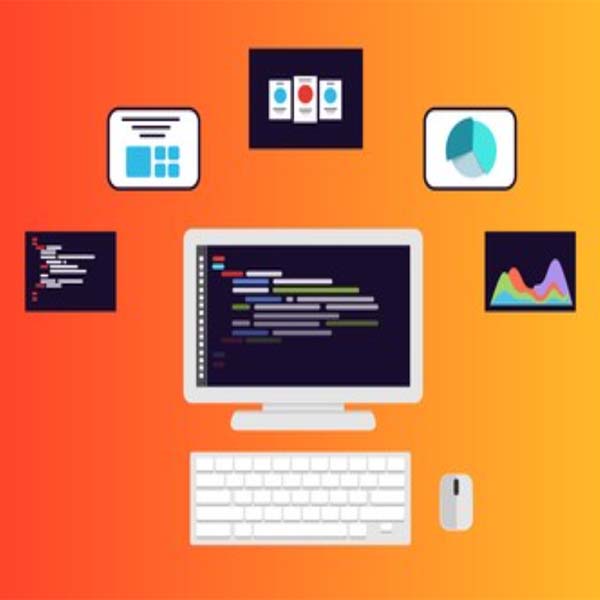
About Course
Unlock your potential as a web developer with this comprehensive Advanced Web Developer Bootcamp, completely **free** on Theetay! This course, taught by professional bootcamp instructors, is packed with the latest technologies, tools, and libraries to take your skills to the next level. Learn from the expertise shared with hundreds of students in person and hundreds of thousands online.
This course, available on platforms like Udemy, Udacity, Coursera, MasterClass, NearPeer, and more, covers a vast range of essential topics:
- Advanced CSS, Advanced JavaScript, NodeJS, D3, React, and Redux
- CSS3 Transitions, Transforms, and Animations
- Testing with Jasmine
- D3, SVG, building charts, force graphs, and data visualizations
- ES2015 keywords, arrow functions, class syntax, and much more
- ES2016 and ES2017, cutting-edge features to JavaScript
- Callbacks, Promises, Generators, and Async Functions
- Building Node.js APIs and Single Page Applications
- Object Oriented Programming in JavaScript
- Closures and the keyword ‘this’
- Functional Programming in JavaScript
- Authentication and Authorization
- Asynchronous Code with JavaScript
This course goes beyond traditional online courses by offering:
- Lectures
- Code-Alongs
- Projects
- Exercises and Solutions
- Research Assignments
- Slides
- Downloads
- Readings
Start your journey to becoming a modern JavaScript web developer with this comprehensive and engaging bootcamp. Enroll today and discover the exciting world of web development!
What Will You Learn?
- Make REAL web applications using cutting-edge technologies
- Build responsive applications using modern CSS technologies like flexbox
- Build JSON APIs using Node, Express and MongoDB
- Learn the most popular front end library React and master the fundamentals around state, props and the component lifecycle
- Use babel and webpack to transpile and bundle code
- Understand what the Virtual DOM is and how React performs reconciliation
- Leverage Component Lifecycle methods with React to include making AJAX calls properly
- Secure Node, Express, Mongo and React applications on the front-end and back-end using bcrypt and JSON Web Tokens
- Add routing to a single page application with the most popular routing library for react, React Router.
- Manage State with a centralized store using Redux
- Connect Redux with a React application to build scalable production applications with client-side state management
- Select and manipulate elements in the DOM using D3
- Build dynamic visualizations using D3 and SVG
- Use D3 to build scatterplots, histograms, pie charts and force graphs
- Build compelling map visualizations with GeoJSON and TopoJSON
- Master how to handle asynchronous code using callbacks, promises, generators and async functions
- Understand how JavaScript handles asynchronous code using the Event Loop with the Stack, Heap and Queue.
- Use advanced array methods to build a solid understanding of functional programming
- Create dynamic single page applications using AJAX
- Structure applications with design patterns using closure and modules
- Explain how Object Oriented Programming works with a thorough understanding of the keyword this and the new keyword
- Refactor code using call, apply and bind to remove duplication
- Use jQuery to build single page applications and understand the limitations of just using jQuery
- Create block scope with the let keyword and use the const keyword to prevent variables from being redeclared
- Clean up code using arrow functions and master method binding without using the bind keyword
- Use default parameters, for of loops and the rest and spread operator to write more concise and more maintainable code
- Refactor an ES5 application to use ES2015
- Master the new class syntax and create instance methods, class methods
- Implement inheritance in ES2015 using extends and super
- Leverage newer data structures like Maps and Sets to better solve problems
- Learn cutting edge features to the JavaScript language with ES2016, 2017 as well as experimental JavaScript additions
- Solve problems similar to what you would get in a developer interview or phone screen
Course Content
01 Course Introduction
-
A Message from the Professor
-
001 The Story Of This Course.mp4
00:00 -
002 Curriculum Walkthrough.mp4
00:00 -
003 Learning Paths.mp4
00:00 -
004 Introducing the Team.mp4
00:00
02 CSS Animations Transforms and Transitions
-
005 Section Introduction.mp4
00:00 -
006 Introduction To CSS Animations.mp4
00:00 -
007 Why Animations Matter.mp4
00:00 -
008 Intro To Pseudoclasses.mp4
00:00 -
009 Pseudo-Classes Hover.mp4
00:00 -
010 Pseudo-Classes Focus.mp4
00:00 -
011 Pseudo-Classes Active.mp4
00:00 -
012 Quick Pseudoclasses Exercise.html
00:00 -
013 Building An Animated Button.mp4
00:00 -
014 Introduction to Transform.mp4
00:00 -
015 Transform Translate.mp4
00:00 -
016 TransformScale() and Transform-Origin.mp4
00:00 -
017 Transform Rotate().mp4
00:00 -
018 A Note on Vendor Prefixes.mp4
00:00 -
019 Transitions Basics.mp4
00:00 -
020 Transition-Duration and Transition-Property.mp4
00:00 -
021 Transition-Timing-Function and Transition-Delay.mp4
00:00 -
022 Transition Shorthand.mp4
00:00 -
023 CSS Animation Performance.mp4
00:00 -
024 Building An Animated Gallery.mp4
00:00
03 CSS Animations Keyframes
-
025 Introduction to Keyframes.mp4
00:00 -
026 Codealong Animated Rainbow Flashing Text.mp4
00:00 -
027 Other CSS Animation Properties.mp4
00:00 -
028 Exercise Rising and Setting Sun Animation.mp4
00:00 -
029 Exercise SOLUTION Rising and Setting Sun Animation.mp4
00:00 -
030 CSS Animation Shorthand.mp4
00:00 -
031 Building an Animated CSS Loading Icon.mp4
00:00
04 Advanced CSS Layout With Flexbox
-
032 Section Introduction.mp4
00:00 -
033 Introduction To Flexbox.mp4
00:00 -
034 The Magic of Display Flex.mp4
00:00 -
035 Important Flexbox Terminology.mp4
00:00 -
036 Flex-Direction.mp4
00:00 -
037 Flex-Wrap.mp4
00:00 -
038 Justify-Content.mp4
00:00 -
039 Flexbox Sidebar Exercise.mp4
00:00 -
040 Flexbox Sidebar Exercise SOLUTION.mp4
00:00 -
041 Align-Items.mp4
00:00 -
042 Align-Content.mp4
00:00 -
043 Building A Responsive Navbar CODEALONG.mp4
00:00 -
044 Align-Self.mp4
00:00 -
045 Order.mp4
00:00 -
046 flex-basis.mp4
00:00 -
047 flex-grow.mp4
00:00 -
048 Flex-Shrink.mp4
00:00 -
049 Building a Polygon.com Widget.mp4
00:00 -
050 Exercise Holy Grail Layout.mp4
00:00 -
051 Exercise Holy Grail Layout SOLUTION.mp4
00:00 -
052 Flexbox Browser Support.mp4
00:00
05 Project Building A Startup Site
-
053 Introducing Matt.mp4
00:00 -
054 Section Introduction.mp4
00:00 -
055 Project Introduction.mp4
00:00 -
056 Project Solution Nav and Header.mp4
00:00 -
057 Project Solution Destinations and Features.mp4
00:00 -
058 Project Solution Testimonials Contact and Footer.mp4
00:00 -
059 Project Solution Responsive Design.mp4
00:00
06 Async Foundations
-
060 Introducing Tim.mp4
00:00 -
061 Introduction.mp4
00:00 -
062 Callback Functions.mp4
00:00 -
063 Codealong forEach.mp4
00:00 -
064 findIndex Exercise Intro.mp4
00:00 -
065 findIndex Solution.mp4
00:00 -
066 The Stack And The Heap.mp4
00:00 -
067 The Stack An Example.mp4
00:00 -
068 setTimeout and setInterval.mp4
00:00 -
069 countDown Exercise Solution.mp4
00:00 -
070 The Event Loop And The Queue.mp4
00:00 -
071 Promise Basics.mp4
00:00 -
072 Promise Chaining.mp4
00:00
07 AJAX Part 1 XHR and Fetch
-
073 Intro to AJAX.mp4
00:00 -
074 Whats the deal with JSON and XML.mp4
00:00 -
075 Making Our First Request with XMLHTTPRequest.mp4
00:00 -
076 AJAX Workflow Building The Random Image App.mp4
00:00 -
077 Bitcoin Price Exercise.mp4
00:00 -
078 Bitcoin Price Exercise Solution.mp4
00:00 -
079 Fetch Introduction.mp4
00:00 -
080 Fetch Options.mp4
00:00 -
081 Fetch Error Handling.mp4
00:00 -
082 Fetch Random User Profile Exercise.mp4
00:00 -
083 Fetch Random User Profile Exercise Solution.mp4
00:00 -
084 The Problem With Fetch.mp4
00:00
08 AJAX Part 2 jQuery and Axios
-
085 jQuery AJAX Introduction.mp4
00:00 -
086 jQuery .ajax Method.mp4
00:00 -
087 Digging In The jQuery Sourcecode.mp4
00:00 -
088 jQuery AJAX Shorthand Methods.mp4
00:00 -
089 jQuery Random Cats API Exercise.mp4
00:00 -
090 jQuery Random Cats Exercise SOLUTION.mp4
00:00 -
091 Axios Intro.mp4
00:00 -
092 Axios Error Handling.mp4
00:00 -
093 Ron Swanson Exercise.mp4
00:00 -
094 Ron Swanson Exercise Solution.mp4
00:00
09 Testing With Jasmine
-
095 Introducing Elie.mp4
00:00 -
096 Section Introduction.mp4
00:00 -
097 Writing Tests in the Browser.mp4
00:00 -
098 Jasmine Syntax and Matchers.mp4
00:00 -
099 Writing Better tests with Hooks.mp4
00:00 -
100 Spies.mp4
00:00 -
101 Clocks.mp4
00:00 -
102 TDD and BDD.mp4
00:00 -
103 Different Types of Tests.mp4
00:00
10 Advanced Array Methods
-
104 Section Introduction.mp4
00:00 -
105 forEach.mp4
00:00 -
106 Exercise SOLUTION forEach.mp4
00:00 -
107 map.mp4
00:00 -
108 Exercise SOLUTION Map.mp4
00:00 -
109 Filter.mp4
00:00 -
110 Exercise SOLUTION Filter.mp4
00:00 -
111 Some.mp4
00:00 -
112 Every.mp4
00:00 -
113 Exercise SOLUTION Some and Every.mp4
00:00 -
114 Reduce.mp4
00:00 -
115 Reduce Continued.mp4
00:00 -
116 Exercise SOLUTION Reduce.mp4
00:00 -
117 Array Methods Recap.mp4
00:00
11 Closures and the Keyword this
-
118 Section Introduction.mp4
00:00 -
119 Introduction to Closures.mp4
00:00 -
120 Using Closures in the Wild.mp4
00:00 -
121 Exercise SOLUTION Closures.mp4
00:00 -
122 Closures Recap.mp4
00:00 -
123 Introduction to the Keyword this.mp4
00:00 -
124 this with Functions and use strict.mp4
00:00 -
125 ObjectImplicit Binding.mp4
00:00 -
126 Explicit Binding.mp4
00:00 -
127 Call.mp4
00:00 -
128 Apply.mp4
00:00 -
129 Bind.mp4
00:00 -
130 Bind Continued.mp4
00:00 -
131 Exercise SOLUTIONS Call Apply Bind.mp4
00:00 -
132 The new Keyword and section recap.mp4
00:00
12 Object Oriented Programming with JavaScript
-
133 Section Introduction.mp4
00:00 -
134 Introduction to Object Oriented Programming with JavaScript.mp4
00:00 -
135 The new Keyword.mp4
00:00 -
136 Refactoring with Multiple Constructors.mp4
00:00 -
137 Constructor Functions Recap.mp4
00:00 -
138 Exercise SOLUTION Constructor Functions.mp4
00:00 -
139 Introduction to Prototypes.mp4
00:00 -
140 The Prototype Chain.mp4
00:00 -
141 Adding Methods to the Prototype.mp4
00:00 -
142 Exercise SOLUTIONS Prototypes.mp4
00:00 -
143 Prototypal Inheritance.mp4
00:00 -
144 Exercise SOLUTIONS Inheritance.mp4
00:00 -
145 Object Oriented Programming Recap.mp4
00:00
13 Creating JSON APIs With Node and Mongo
-
146 Section Introduction.mp4
00:00 -
147 Preparing For React.mp4
00:00 -
148 Defining Our API Gameplan.mp4
00:00 -
149 SUPER IMPORTANT UPDATED C9 INSTALLATION INSTRUCTIONS.html
00:00 -
150 Installing NodeJS.mp4
00:00 -
151 Cloud9 Without A Credit Card Instructions.html
00:00 -
152 Installing Node Locally.html
00:00 -
153 Creating Our Initial Express Application.mp4
00:00 -
154 Responding With JSON.mp4
00:00 -
155 Installing Mongo.mp4
00:00 -
156 Defining Our Schema.mp4
00:00 -
157 Defining The Index Route.mp4
00:00 -
158 Defining The Create Route.mp4
00:00 -
159 Defining The Show Route.mp4
00:00 -
160 Defining the Update Route.mp4
00:00 -
161 Defining the Delete Route.mp4
00:00 -
162 Refactoring Our API.mp4
00:00
14 Codealong Single Page Todo List with Express Mongo and jQuery
-
163 Introducing Our Single Page App.mp4
00:00 -
164 Serving Static Files and Nodemon.mp4
00:00 -
165 Adding jQuery and The Starter CSS.mp4
00:00 -
166 Writing The Initial AJAX Call.mp4
00:00 -
167 Displaying Our Todos Correctly.mp4
00:00 -
168 Connecting the Form to our API.mp4
00:00 -
169 Making the Delete Button Work.mp4
00:00 -
170 Toggling Todo Completion.mp4
00:00
15 ESE5 Part I
-
171 Section Introduction.mp4
00:00 -
172 Introduction to ESE5.mp4
00:00 -
173 Const.mp4
00:00 -
174 Let.mp4
00:00 -
175 Template Strings.mp4
00:00 -
176 Introduction to Arrow Functions.mp4
00:00 -
177 Arrow Functions Continued.mp4
00:00 -
178 Exercise SOLUTION Arrow Functions.mp4
00:00 -
179 Default Parameters.mp4
00:00 -
180 For…of Loops.mp4
00:00 -
181 Rest.mp4
00:00 -
182 Spread.mp4
00:00 -
183 Exercise SOLUTION Rest and Spread.mp4
00:00 -
184 Object Enhancements.mp4
00:00 -
185 Object Destructuring.mp4
00:00 -
186 Array Destructuring.mp4
00:00 -
187 Exercise SOLUTION Destructuring.mp4
00:00 -
188 ESE5 Part I Recap.mp4
00:00
16 ESE5 Project – Guess the Password
-
189 Section Introduction.mp4
00:00 -
190 Introduction to Guess the Password.mp4
00:00 -
191 Guess the Password Code Walkthrough.mp4
00:00 -
192 Guess the Password Refactor.mp4
00:00
17 ESE5 Part II
-
193 Section Introduction.mp4
00:00 -
194 Introduction to the class Keyword.mp4
00:00 -
195 Instance Methods.mp4
00:00 -
196 Class Methods.mp4
00:00 -
197 Class Solutions.mp4
00:00 -
198 Inheritance with ESE5.mp4
00:00 -
199 Super.mp4
00:00 -
200 Exercise SOLUTION Inheritance and Super.mp4
00:00 -
201 ESE5 class Keyword Recap.mp4
00:00 -
202 Maps.mp4
00:00 -
203 Sets.mp4
00:00 -
204 Exercise SOLUTION Maps and Sets.mp4
00:00 -
205 Promises.mp4
00:00 -
206 Promises Continued.mp4
00:00 -
207 ESE5 Promises Assignment.html
00:00 -
208 Exercise SOLUTION Promises.mp4
00:00 -
209 Generators.mp4
00:00 -
210 Object.assign and Array.from.mp4
00:00 -
211 Additional Helpful ESE5 Methods.mp4
00:00 -
212 Exercise SOLUTION ESE5 Methods.mp4
00:00
18 ESE6 and ESE7
-
213 Section Introduction.mp4
00:00 -
214 ESE6 Exponentiation Operator and Includes.mp4
00:00 -
215 padStart and padEnd.mp4
00:00 -
216 Async Functions Introduction.mp4
00:00 -
217 Async Functions Continued.mp4
00:00 -
218 Coding Exercise – Async Functions Assignment.html
00:00 -
219 Exercise SOLUTION Async Functions.mp4
00:00 -
220 Object Rest and Spread Recap.mp4
00:00
19 D3 and the DOM
-
221 Section Introduction.mp4
00:00 -
222 An Introduction to D3.mp4
00:00 -
223 D3 Selections.mp4
00:00 -
224 Selections and Callbacks.mp4
00:00 -
225 Event Listeners in D3.mp4
00:00 -
226 Exercise Guess the Password Refactor.html
00:00 -
227 Solution Guess the Password Refactor.mp4
00:00 -
228 Exercise Notes App.mp4
00:00 -
229 Solution Notes App.mp4
00:00
20 Data Joins and Update Patterns in D3
-
230 Section Introduction.mp4
00:00 -
231 Basic Data Joins and Enter Selections.mp4
00:00 -
232 Exit Selections and Key Functions.mp4
00:00 -
233 The General Update Pattern in D3.mp4
00:00 -
234 Exercise Character Frequencies.mp4
00:00 -
235 Solution Character Frequencies.mp4
00:00
21 SVG and D3
-
236 Section Introduction.mp4
00:00 -
237 Introduction to SVG.mp4
00:00 -
238 Rectangles Polygons and Circles in SVG.mp4
00:00 -
239 Text Elements in SVG.mp4
00:00 -
240 Path Elements in SVG.mp4
00:00 -
241 Exercise SVG Flags.mp4
00:00 -
242 Solution SVG Flags.mp4
00:00 -
243 Introduction to SVG and D3.mp4
00:00 -
244 Exercise Character Frequencies Revisited.mp4
00:00 -
245 Solution Character Frequencies Revisited.mp4
00:00
22 Intermediate D3
-
246 Section Introduction.mp4
00:00 -
247 Extrema and Scales.mp4
00:00 -
248 Scatterplots.mp4
00:00 -
249 Axes and Gridlines.mp4
00:00 -
250 Exercise Scatterplot.mp4
00:00 -
251 Solution Scatterplot.mp4
00:00 -
252 Histograms.mp4
00:00 -
253 Histograms Continued.mp4
00:00 -
254 Exercise Histograms.mp4
00:00 -
255 Solution Histograms.mp4
00:00 -
256 Pie Charts.mp4
00:00 -
257 Pie Charts Continued.mp4
00:00 -
258 Exercise Pie Charts.mp4
00:00 -
259 Solution Pie Charts.mp4
00:00
23 D3 Odds and Ends and Advanced Graph Types
-
260 Section Introduction.mp4
00:00 -
261 Tooltips.mp4
00:00 -
262 Transitions.mp4
00:00 -
263 Managing Asynchronous Code with D3.mp4
00:00 -
264 Exercise D3 Odds and Ends.mp4
00:00 -
265 Solution D3 Odds and Ends.mp4
00:00 -
266 An Introduction to GeoJSON.mp4
00:00 -
267 An Introduction to TopoJSON.mp4
00:00 -
268 Map Visualization Example.mp4
00:00 -
269 Nodes in Force-Directed Graphs.mp4
00:00 -
270 Links in Force-Directed Graphs.mp4
00:00 -
271 Dragging Nodes and Alpha Values.mp4
00:00 -
272 General Update Pattern with Force-Directed Graphs.mp4
00:00
24 Project Building a Data Dashboard with D3
-
273 Project Introduction and Requirements.mp4
00:00 -
274 Project Example Solution Part 1.mp4
00:00 -
275 Project Example Solution Part 2.mp4
00:00
25 Introduction To React and JSX
-
276 START HERE FOR REACT.html
00:00 -
277 Section Introduction.mp4
00:00 -
278 How To Get Our React Solution Code.html
00:00 -
279 Front-end Framework Introduction.mp4
00:00 -
280 First React Component.mp4
00:00 -
281 JSX.mp4
00:00 -
282 JSX With JavaScript.mp4
00:00 -
283 Exercise Random Box.mp4
00:00 -
284 Random Box Assignment Solution.mp4
00:00 -
285 Multiple React Components.mp4
00:00
26 Create React App and Props
-
286 Create React App.mp4
00:00 -
287 Create React App Files.mp4
00:00 -
288 JavaScript Import Statements.mp4
00:00 -
289 Import HobbyList Assignment.mp4
00:00 -
290 Import HobbyList Solution.mp4
00:00 -
291 Intro to Props.mp4
00:00 -
292 Recipe App With Props.mp4
00:00 -
293 Recipe App With Props Continued.mp4
00:00 -
294 Default Props and Prop Types.mp4
00:00 -
295 Recipe App Props Exercise.mp4
00:00 -
296 Recipe App Props Solution.mp4
00:00 -
297 props.children.mp4
00:00
27 State
-
298 Section Introduction.mp4
00:00 -
299 Intro to State.mp4
00:00 -
300 Pure Functions.mp4
00:00 -
301 Update Complex State Exercise.mp4
00:00 -
302 Update Complex State Solution.mp4
00:00 -
303 React Component Architecture.mp4
00:00 -
304 setState Can Be Tricky.mp4
00:00 -
305 React DevTools.mp4
00:00 -
306 Colored Boxes Exercise.mp4
00:00 -
307 Colored Boxes Solution.mp4
00:00
28 The Virtual DOM Events and Forms
-
308 Section Introduction.mp4
00:00 -
309 The Virtual DOM.mp4
00:00 -
310 Events.mp4
00:00 -
311 Forms.mp4
00:00 -
312 Todo App Exercise.mp4
00:00 -
313 Todo App Solution.mp4
00:00 -
314 Refs.mp4
00:00 -
315 Recipe App With State.mp4
00:00 -
316 Recipe App With State – New Recipe Form.mp4
00:00 -
317 Recipe App With State – Saving The New Recipe.mp4
00:00 -
318 Recipe App With State – ShowHide Form.mp4
00:00 -
319 Recipe App With State – Delete Recipe.mp4
00:00 -
320 Memory Game.mp4
00:00 -
321 Memory Game Solution Part 1.mp4
00:00 -
322 Memory Game Solution Part 2.mp4
00:00
29 Component Lifecycle Methods
-
323 Introduction To Component Lifecycle Methods.mp4
00:00 -
324 Component Lifecycle Method Examples.mp4
00:00 -
325 Country Flag Guessing App Exercise.mp4
00:00 -
326 Country Flag Guessing App Solution.mp4
00:00
30 Building A Full-Stack App With React
-
327 Setting Up Our ExpressMongo API.mp4
00:00 -
328 Creating Our React App.mp4
00:00 -
329 Adding the TodoList Component.mp4
00:00 -
330 Loading Initial Data from API.mp4
00:00 -
331 Creating the TodoItem Component.mp4
00:00 -
332 Adding the TodoForm Component.mp4
00:00 -
333 Deleting Todos.mp4
00:00 -
334 Toggling Todos.mp4
00:00 -
335 Refactoring API Calls with Async Functions.mp4
00:00
31 React Router
-
336 Section Introduction.mp4
00:00 -
337 HTML5 History Object.mp4
00:00 -
338 Introduction to React Router.mp4
00:00 -
339 Introduction to React Router Continued.mp4
00:00
32 Redux Introduction
-
340 Section Introduction.mp4
00:00 -
341 Introduction to Redux Without React.mp4
00:00 -
342 React With Redux.mp4
00:00 -
343 Organizing Redux.mp4
00:00
33 In Depth Redux Code Walkthrough
-
344 Redux Intro.mp4
00:00 -
345 Redux Counter.mp4
00:00 -
346 Redux Todos.mp4
00:00 -
347 Redux React Intro.mp4
00:00 -
348 Redux React Continued.mp4
00:00 -
349 Redux React mapDispatchToProps.mp4
00:00 -
350 React Router Redux.mp4
00:00 -
351 Todos Node Backend.mp4
00:00 -
352 Full Stack Redux.mp4
00:00
34 Warbler Introduction and Backend Part 1
-
353 Warbler Intro.mp4
00:00 -
354 Introduction to Authentication.mp4
00:00 -
355 Getting Started with the Backend.mp4
00:00 -
356 Adding an Error Handler.mp4
00:00 -
357 Adding a User Model.mp4
00:00 -
358 User Middleware.mp4
00:00 -
359 Storing Passwords Properly.mp4
00:00 -
360 Section Review.mp4
00:00
35 Warbler Backend Part 2
-
361 Adding Signin Functionality.mp4
00:00 -
362 Messages Intro.mp4
00:00 -
363 Creating a message.mp4
00:00 -
364 Adding loginRequired middleware.mp4
00:00 -
365 Adding ensureCorrectUser middleware.mp4
00:00 -
366 Adding additional messages routes.mp4
00:00 -
367 Testing with HTTPie and Review.mp4
00:00
36 Warbler Frontend Part 1
-
368 Warbler Frontend Introduction.mp4
00:00 -
369 Reducers – errors and currentUser.mp4
00:00 -
370 Warbler Redux Store setup.mp4
00:00 -
371 Adding a Navbar.mp4
00:00 -
372 Styling the Navbar Component.mp4
00:00 -
373 Warbler Main Component.mp4
00:00 -
374 Styling the Homepage Component.mp4
00:00 -
375 Warbler Authform Part 1.mp4
00:00 -
376 Warbler Authform Part 2.mp4
00:00 -
377 Warbler Authform Part 3.mp4
00:00 -
378 Warbler Frontend Section Review.mp4
00:00
37 Warbler Frontend Part 2
-
379 Handling Error Messages with Redux.mp4
00:00 -
380 Redirecting with React Router and Redux.mp4
00:00 -
381 Logging out a User.mp4
00:00 -
382 Adding a JWT to HTTP Headers.mp4
00:00 -
383 Displaying Messages.mp4
00:00 -
384 Message List Component.mp4
00:00 -
385 Higher Order Components for Authentication.mp4
00:00 -
386 Adding a new Message.mp4
00:00 -
387 Deleting a Message.mp4
00:00 -
388 Displaying the correct Delete Message button.mp4
00:00 -
389 Additional Styling and Section Review.mp4
00:00 -
390 Deploying the Backend.mp4
00:00 -
391 Deploying the Frontend.mp4
00:00
Earn a certificate
Add this certificate to your resume to demonstrate your skills & increase your chances of getting noticed.
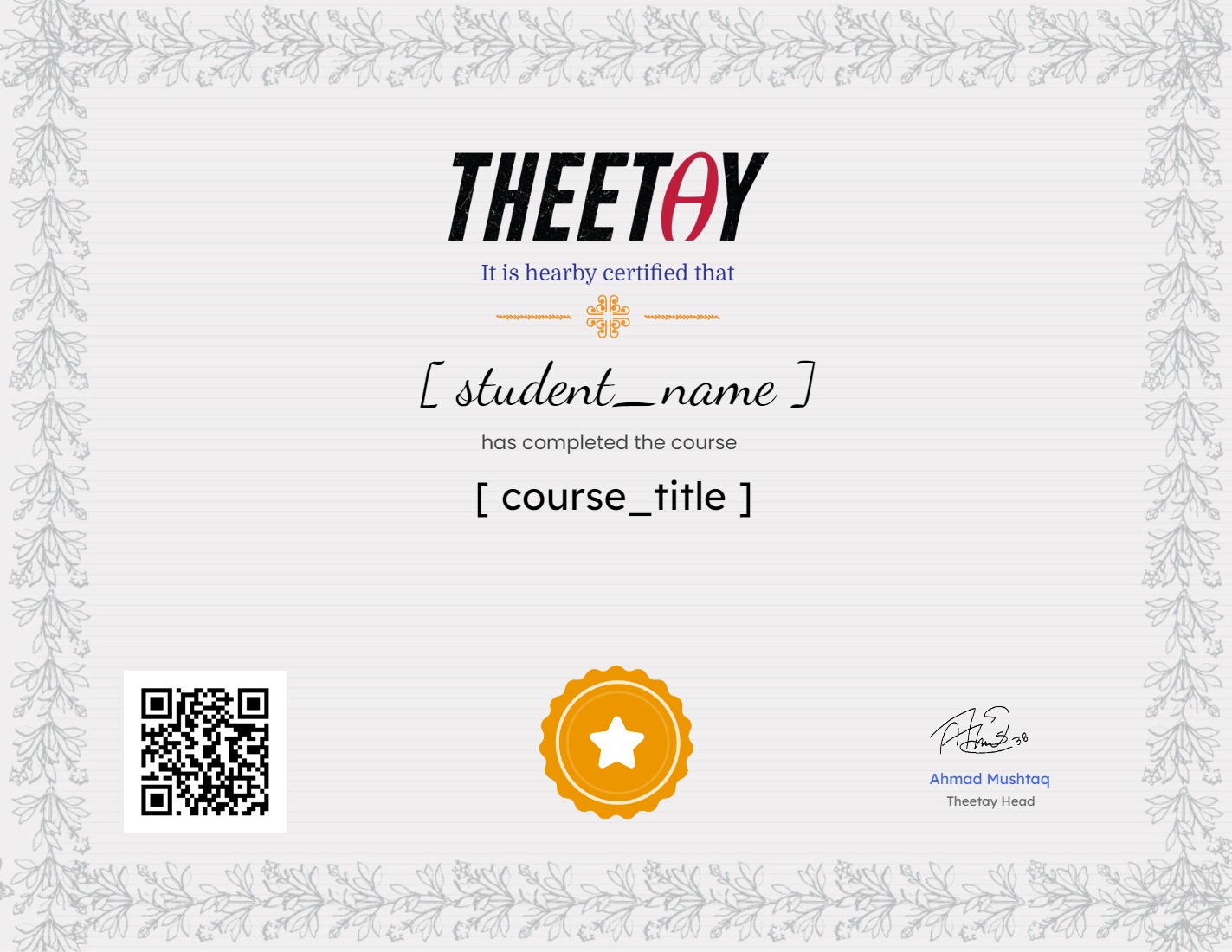