Node.js, Express, MongoDB & More: The Complete Bootcamp
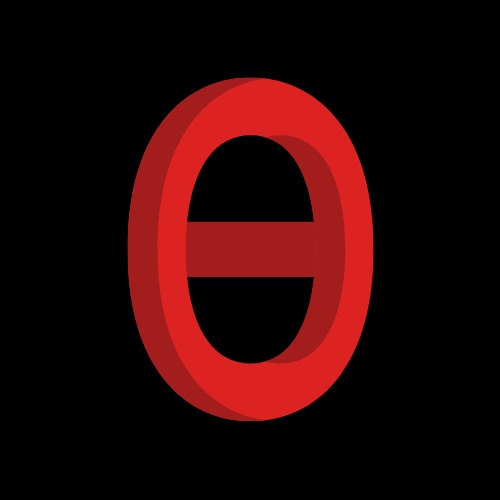
About Course
Learn Node.js, Express, MongoDB & More: The Complete Bootcamp – FREE
This comprehensive Node.js Bootcamp will transform you from a beginner to a skilled back-end developer. Master modern technologies like Node.js, Express, and MongoDB to build fast and powerful applications. This free course is packed with over 40 hours of high-quality content, including a complete project-based learning experience.
Learn through hands-on projects and build a real-world, feature-rich application with a RESTful API and server-side rendered website. This course covers all aspects of modern back-end development, from fundamentals to advanced concepts.
What You Will Learn:
- Node.js fundamentals, core modules, and NPM
- Node.js internals: event loop, blocking/non-blocking code, event-driven architecture, streams, modules
- Express fundamentals: routing, middleware, sending responses
- RESTful API design and development with advanced features: filtering, sorting, aliasing, pagination
- Server-side website rendering (HTML) with Pug templates
- CRUD operations with MongoDB database (local and cloud-based)
- Advanced MongoDB: geospatial queries, aggregation pipeline, operators
- Mongoose fundamentals: data models, CRUD operations, validation, middleware
- Advanced Mongoose features: modeling geospatial data, populates, virtual populates, indexes
- MVC (Model-View-Controller) architecture
- NoSQL database data handling
- Advanced data modeling: relationships, embedding, referencing
- Complete modern authentication with JWT: sign up, log in, password reset, secure cookies
- Authorization (user roles)
- Security best practices: encryption, sanitization, rate limiting
- Credit card payment processing with Stripe
- File uploading and image processing
- Email sending with Mailtrap and Sendgrid
- Advanced error handling workflows
- Deploying Node.js applications with Heroku
- Git and GitHub crash course
- And much more!
Why Learn Node.js?
Node.js is a popular and in-demand technology used by major companies like Netflix, PayPal, and Uber. It allows you to build powerful back-end applications using your existing JavaScript knowledge, making your full-stack development process easier and faster. Learn Node.js today and become a more versatile and sought-after developer.
Why Choose This Course?
This is the biggest Node.js course available, offering the most comprehensive project and in-depth explanations. Even if you have some Node.js experience, this course will expose you to advanced topics not covered elsewhere.
Free Access & Course Benefits:
This course is completely **free** to access. You’ll enjoy:
- Lifetime access to 40+ hours of HD quality videos
- Friendly and responsive support in the course Q&A
- English closed captions
- Course slides in PDF format
- Downloadable assets, starter code, and final code for each section
- Small challenges to track your progress
Start learning Node.js for FREE today. Enroll now and join this incredible journey!
**Note:** This course is designed for individuals with basic JavaScript knowledge. No prior back-end experience is required.
**Available on:** Udemy, Udacity, Coursera, MasterClass, NearPeer, and other platforms.
What Will You Learn?
- Master the entire modern back-end stack: Node, Express, MongoDB and Mongoose (MongoDB JS driver)
- Build a complete, beautiful & real-world application from start to finish (API and server-side rendered website)
- Build a fast, scalable, feature-rich RESTful API (includes filters, sorts, pagination, and much more)
- Learn how Node really works behind the scenes: event loop, blocking vs non-blocking code, streams, modules, etc.
- CRUD operations with MongoDB and Mongoose
- Deep dive into mongoose (including all advanced features)
- How to work with data in NoSQL databases (including geospatial data)
- Advanced authentication and authorization (including password reset)
- Security: encryption, sanitization, rate limiting, etc.
- Server-side website rendering with Pug templates
- Credit card payments with Stripe
- Sending emails & uploading files
- Deploy the final application to production (including a Git crash-course)
- Downloadable videos, code and design assets for projects
Course Content
01 – Welcome, Welcome, Welcome!
-
001 Course Structure and Projects.mp4
00:00 -
002 READ BEFORE YOU START!.html
00:00 -
003 Let’s Install Node.js.mp4
00:00 -
Section Quiz
02 – Introduction to Node.js and NPM
-
001 Section Intro.mp4
00:00 -
002 What Is Node.js and Why Use It.mp4
00:00 -
003 Running Javascript Outside the Browser.mp4
00:00 -
004 Using Modules 1 Core Modules.mp4
00:00 -
005 Reading and Writing Files.mp4
00:00 -
006 Blocking and Non-Blocking Asynchronous Nature of Node.js.mp4
00:00 -
007 Reading and Writing Files Asynchronously.mp4
00:00 -
008 Creating a Simple Web Server.mp4
00:00 -
009 Routing.mp4
00:00 -
010 Building a (Very) Simple API.mp4
00:00 -
011 HTML Templating Building the Templates.mp4
00:00 -
012 HTML Templating Filling the Templates.mp4
00:00 -
013 Parsing Variables from URLs.mp4
00:00 -
014 Using Modules 2 Our Own Modules.mp4
00:00 -
015 Introduction to NPM and the package.json File.mp4
00:00 -
016 Types of Packages and Installs.mp4
00:00 -
017 Using Modules 3 3rd Party Modules.mp4
00:00 -
018 Package Versioning and Updating.mp4
00:00 -
019 Setting up Prettier in VS Code.mp4
00:00 -
020 Recap and What’s Next.mp4
00:00 -
external-links.txt
00:00 -
Section Quiz
03 – Introduction to Back-End Web Development
-
001 Section Intro.mp4
00:00 -
002 An Overview of How the Web Works.mp4
00:00 -
003 HTTP in Action.mp4
00:00 -
004 Front-End vs. Back-End Web Development.mp4
00:00 -
005 Static vs Dynamic vs API.mp4
00:00 -
Section Quiz
04 – How Node.js Works A Look Behind the Scenes
-
001 Section Intro.mp4
00:00 -
002 Node, V8, Libuv and C++.mp4
00:00 -
003 Processes, Threads and the Thread Pool.mp4
00:00 -
004 The Node.js Event Loop.mp4
00:00 -
005 The Event Loop in Practice.mp4
00:00 -
006 Events and Event-Driven Architecture.mp4
00:00 -
007 Events in Practice.mp4
00:00 -
008 Introduction to Streams.mp4
00:00 -
009 Streams in Practice.mp4
00:00 -
010 How Requiring Modules Really Works.mp4
00:00 -
011 Requiring Modules in Practice.mp4
00:00 -
external-links.txt
00:00 -
Section Quiz
05 – (Optional) Asynchronous JavaScript Promises and AsyncAwait
-
001 Section Intro.mp4
00:00 -
002 The Problem with Callbacks Callback Hell.mp4
00:00 -
003 From Callback Hell to Promises.mp4
00:00 -
004 Building Promises.mp4
00:00 -
005 Consuming Promises with AsyncAwait.mp4
00:00 -
006 Returning Values from Async Functions.mp4
00:00 -
007 Waiting for Multiple Promises Simultaneously.mp4
00:00 -
external-links.txt
00:00 -
Section Quiz
06 – Express Let’s Start Building the Natours API!
-
001 Section Intro.mp4
00:00 -
002 What is Express.mp4
00:00 -
003 Installing Postman.mp4
00:00 -
004 Setting up Express and Basic Routing.mp4
00:00 -
005 APIs and RESTful API Design.mp4
00:00 -
006 Starting Our API Handling GET Requests.mp4
00:00 -
007 Handling POST Requests.mp4
00:00 -
008 Responding to URL Parameters.mp4
00:00 -
009 Handling PATCH Requests.mp4
00:00 -
010 Handling DELETE Requests.mp4
00:00 -
011 Refactoring Our Routes.mp4
00:00 -
012 Middleware and the Request-Response Cycle.mp4
00:00 -
013 Creating Our Own Middleware.mp4
00:00 -
014 Using 3rd-Party Middleware.mp4
00:00 -
015 Implementing the Users Routes.mp4
00:00 -
016 Creating and Mounting Multiple Routers.mp4
00:00 -
017 A Better File Structure.mp4
00:00 -
018 Param Middleware.mp4
00:00 -
019 Chaining Multiple Middleware Functions.mp4
00:00 -
020 Serving Static Files.mp4
00:00 -
021 Environment Variables.mp4
00:00 -
022 Setting up ESLint + Prettier in VS Code.mp4
00:00 -
external-links.txt
00:00 -
Section Quiz
07 – Introduction to MongoDB
-
001 Section Intro.mp4
00:00 -
002 What is MongoDB.mp4
00:00 -
003 No Need to Install MongoDB Locally.html
00:00 -
004 (OPTIONAL) Installing MongoDB on macOS.mp4
00:00 -
005 (OPTIONAL) Installing MongoDB on Windows.mp4
00:00 -
006 (OPTIONAL) Creating a Local Database.mp4
00:00 -
007 (OPTIONAL) CRUD Creating Documents.mp4
00:00 -
008 (OPTIONAL) CRUD Querying (Reading) Documents.mp4
00:00 -
009 (OPTIONAL) CRUD Updating Documents.mp4
00:00 -
010 (OPTIONAL) CRUD Deleting Documents.mp4
00:00 -
011 Using Compass App for CRUD Operations.mp4
00:00 -
012 Creating a Hosted Database with Atlas.mp4
00:00 -
013 Connecting to Our Hosted Database.mp4
00:00 -
Section Quiz
08 – Using MongoDB with Mongoose
-
001 Section Intro.mp4
00:00 -
002 Connecting Our Database with the Express App.mp4
00:00 -
003 What Is Mongoose.mp4
00:00 -
004 Creating a Simple Tour Model.mp4
00:00 -
005 Creating Documents and Testing the Model.mp4
00:00 -
006 Intro to Back-End Architecture MVC, Types of Logic, and More.mp4
00:00 -
007 Refactoring for MVC.mp4
00:00 -
008 Another Way of Creating Documents.mp4
00:00 -
009 Reading Documents.mp4
00:00 -
010 Updating Documents.mp4
00:00 -
011 Deleting Documents.mp4
00:00 -
012 Modelling the Tours.mp4
00:00 -
013 Importing Development Data.mp4
00:00 -
014 Making the API Better Filtering.mp4
00:00 -
015 Making the API Better Advanced Filtering.mp4
00:00 -
016 Making the API Better Sorting.mp4
00:00 -
017 Making the API Better Limiting Fields.mp4
00:00 -
018 Making the API Better Pagination.mp4
00:00 -
019 Making the API Better Aliasing.mp4
00:00 -
020 Refactoring API Features.mp4
00:00 -
021 Aggregation Pipeline Matching and Grouping.mp4
00:00 -
022 Aggregation Pipeline Unwinding and Projecting.mp4
00:00 -
023 Virtual Properties.mp4
00:00 -
024 Document Middleware.mp4
00:00 -
025 Query Middleware.mp4
00:00 -
026 Aggregation Middleware.mp4
00:00 -
027 Data Validation Built-In Validators.mp4
00:00 -
028 Data Validation Custom Validators.mp4
00:00 -
Section Quiz
09 – Error Handling with Express
-
001 Section Intro.mp4
00:00 -
002 Debugging Node.js with ndb.mp4
00:00 -
003 Handling Unhandled Routes.mp4
00:00 -
004 An Overview of Error Handling.mp4
00:00 -
005 Implementing a Global Error Handling Middleware.mp4
00:00 -
006 Better Errors and Refactoring.mp4
00:00 -
007 Catching Errors in Async Functions.mp4
00:00 -
008 Adding 404 Not Found Errors.mp4
00:00 -
009 Errors During Development vs Production.mp4
00:00 -
010 Handling Invalid Database IDs.mp4
00:00 -
011 Handling Duplicate Database Fields.mp4
00:00 -
012 Handling Mongoose Validation Errors.mp4
00:00 -
013 Errors Outside Express Unhandled Rejections.mp4
00:00 -
014 Catching Uncaught Exceptions.mp4
00:00 -
Section Quiz
10 – Authentication, Authorization and Security
-
001 Section Intro.mp4
00:00 -
002 Modelling Users.mp4
00:00 -
003 Creating New Users.mp4
00:00 -
004 Managing Passwords.mp4
00:00 -
005 How Authentication with JWT Works.mp4
00:00 -
006 Signing up Users.mp4
00:00 -
007 Logging in Users.mp4
00:00 -
008 Protecting Tour Routes – Part 1.mp4
00:00 -
009 Protecting Tour Routes – Part 2.mp4
00:00 -
010 Advanced Postman Setup.mp4
00:00 -
011 Authorization User Roles and Permissions.mp4
00:00 -
012 Password Reset Functionality Reset Token.mp4
00:00 -
013 Sending Emails with Nodemailer.mp4
00:00 -
014 Password Reset Functionality Setting New Password.mp4
00:00 -
015 Updating the Current User Password.mp4
00:00 -
016 Updating the Current User Data.mp4
00:00 -
017 Deleting the Current User.mp4
00:00 -
018 Security Best Practices.mp4
00:00 -
019 Sending JWT via Cookie.mp4
00:00 -
020 Implementing Rate Limiting.mp4
00:00 -
021 Setting Security HTTP Headers.mp4
00:00 -
022 Data Sanitization.mp4
00:00 -
023 Preventing Parameter Pollution.mp4
00:00 -
Section Quiz
11 – Modelling Data and Advanced Mongoose
-
001 Section Intro.mp4
00:00 -
002 MongoDB Data Modelling.mp4
00:00 -
003 Designing Our Data Model.mp4
00:00 -
004 Modelling Locations (Geospatial Data).mp4
00:00 -
005 Modelling Tour Guides Embedding.mp4
00:00 -
006 Modelling Tour Guides Child Referencing.mp4
00:00 -
007 Populating Tour Guides.mp4
00:00 -
008 Modelling Reviews Parent Referencing.mp4
00:00 -
009 Creating and Getting Reviews.mp4
00:00 -
010 Populating Reviews.mp4
00:00 -
011 Virtual Populate Tours and Reviews.mp4
00:00 -
012 Implementing Simple Nested Routes.mp4
00:00 -
013 Nested Routes with Express.mp4
00:00 -
014 Adding a Nested GET Endpoint.mp4
00:00 -
015 Building Handler Factory Functions Delete.mp4
00:00 -
016 Factory Functions Update and Create.mp4
00:00 -
017 Factory Functions Reading.mp4
00:00 -
018 Adding a me Endpoint.mp4
00:00 -
019 Adding Missing Authentication and Authorization.mp4
00:00 -
020 Importing Review and User Data.mp4
00:00 -
021 Improving Read Performance with Indexes.mp4
00:00 -
022 Calculating Average Rating on Tours – Part 1.mp4
00:00 -
023 Calculating Average Rating on Tours – Part 2.mp4
00:00 -
024 Preventing Duplicate Reviews.mp4
00:00 -
025 Geospatial Queries Finding Tours Within Radius.mp4
00:00 -
026 Geospatial Aggregation Calculating Distances.mp4
00:00 -
027 Creating API Documentation Using Postman.mp4
00:00 -
Section Quiz
12 – Server-Side Rendering with Pug Templates
-
001 Section Intro.mp4
00:00 -
002 Recap Server-Side vs Client-Side Rendering.mp4
00:00 -
003 Setting up Pug in Express.mp4
00:00 -
004 First Steps with Pug.mp4
00:00 -
005 Creating Our Base Template.mp4
00:00 -
006 Including Files into Pug Templates.mp4
00:00 -
007 Extending Our Base Template with Blocks.mp4
00:00 -
008 Setting up the Project Structure.mp4
00:00 -
009 Building the Tour Overview – Part 1.mp4
00:00 -
010 Building the Tour Overview – Part 2.mp4
00:00 -
011 Building the Tour Page – Part 1.mp4
00:00 -
012 Building the Tour Page – Part 2.mp4
00:00 -
013 Including a Map with Mapbox – Part 1.mp4
00:00 -
014 Including a Map with Mapbox – Part 2.mp4
00:00 -
015 Building the Login Screen.mp4
00:00 -
016 Logging in Users with Our API – Part 1.mp4
00:00 -
017 Logging in Users with Our API – Part 2.mp4
00:00 -
018 Logging in Users with Our API – Part 3.mp4
00:00 -
019 Logging out Users.mp4
00:00 -
020 Rendering Error Pages.mp4
00:00 -
021 Building the User Account Page.mp4
00:00 -
022 Updating User Data.mp4
00:00 -
023 Updating User Data with Our API.mp4
00:00 -
024 Updating User Password with Our API.mp4
00:00
13 – Advanced Features Payments, Email, File Uploads
-
001 Section Intro.mp4
00:00 -
002 Image Uploads Using Multer Users.mp4
00:00 -
003 Configuring Multer.mp4
00:00 -
004 Saving Image Name to Database.mp4
00:00 -
005 Resizing Images.mp4
00:00 -
006 Adding Image Uploads to Form.mp4
00:00 -
007 Uploading Multiple Images Tours.mp4
00:00 -
008 Processing Multiple Images.mp4
00:00 -
009 Building a Complex Email Handler.mp4
00:00 -
010 Email Templates with Pug Welcome Emails.mp4
00:00 -
011 Sending Password Reset Emails.mp4
00:00 -
012 Using Sendgrid for Real Emails.mp4
00:00 -
013 Credit Card Payments with Stripe.mp4
00:00 -
014 Integrating Stripe into the Back-End.mp4
00:00 -
015 Processing Payments on the Front-End.mp4
00:00 -
016 Modelling the Bookings.mp4
00:00 -
017 Creating New Bookings on Checkout Success.mp4
00:00 -
018 Rendering a User’s Booked Tours.mp4
00:00 -
019 Finishing the Bookings API.mp4
00:00 -
020 Final Considerations.mp4
00:00 -
Section Quiz
14 – Setting Up Git and Deployment
-
001 Section Intro.mp4
00:00 -
002 Setting Up Git and GitHub.mp4
00:00 -
003 Git Fundamentals.mp4
00:00 -
004 Pushing to GitHub.mp4
00:00 -
005 Preparing Our App for Deployment.mp4
00:00 -
006 Deploying Our App to Heroku.mp4
00:00 -
007 Testing for Secure HTTPS Connections.mp4
00:00 -
008 Responding to a SIGTERM Signal.mp4
00:00 -
009 Implementing CORS.mp4
00:00 -
010 Finishing Payments with Stripe Webhooks.mp4
00:00 -
Section Quiz
15 – That’s It, Everyone!
-
001 Where to Go from Here.mp4
00:00 -
002 My Other Courses + Updates.html
00:00
Earn a certificate
Add this certificate to your resume to demonstrate your skills & increase your chances of getting noticed.
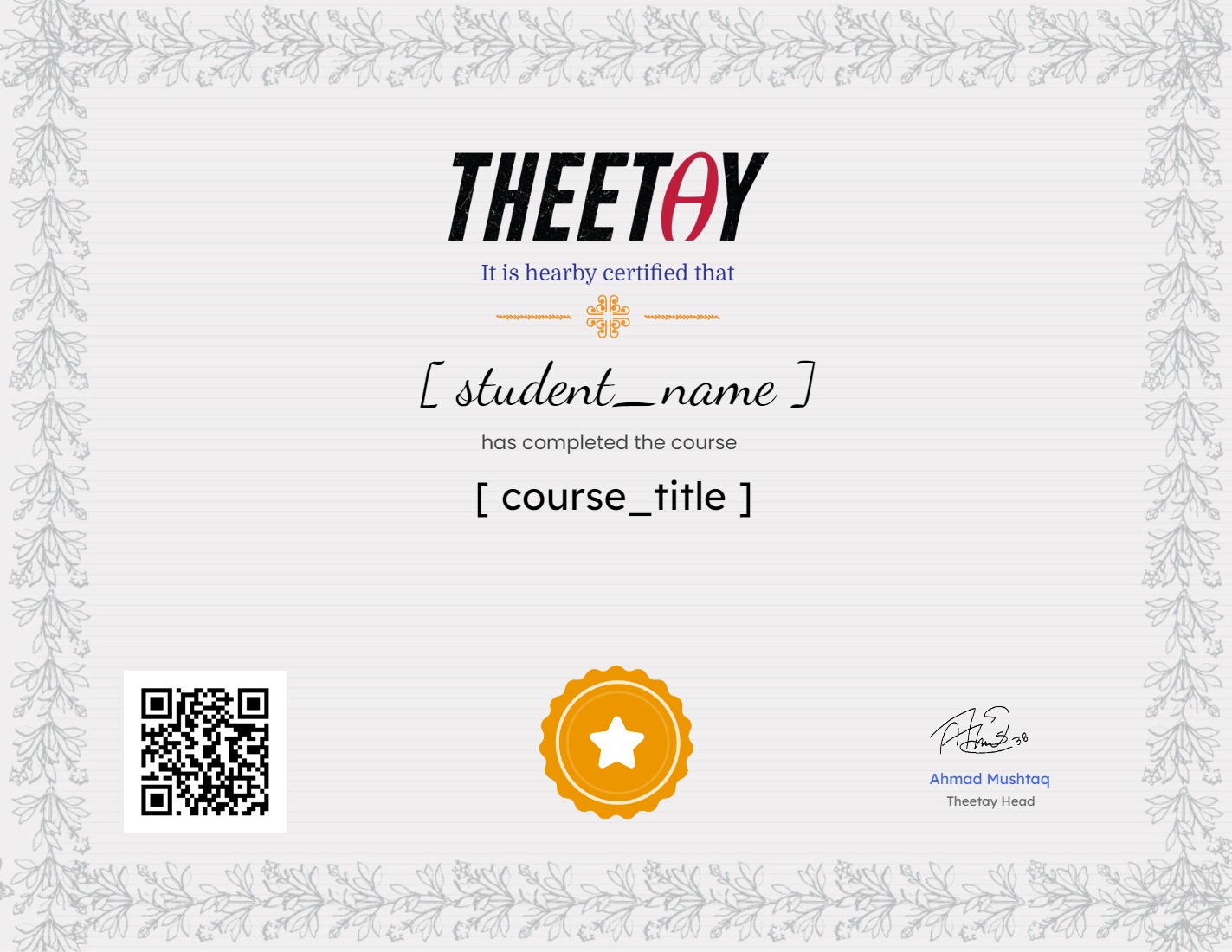