JavaScript – The Complete Guide 2023 (Beginner + Advanced)
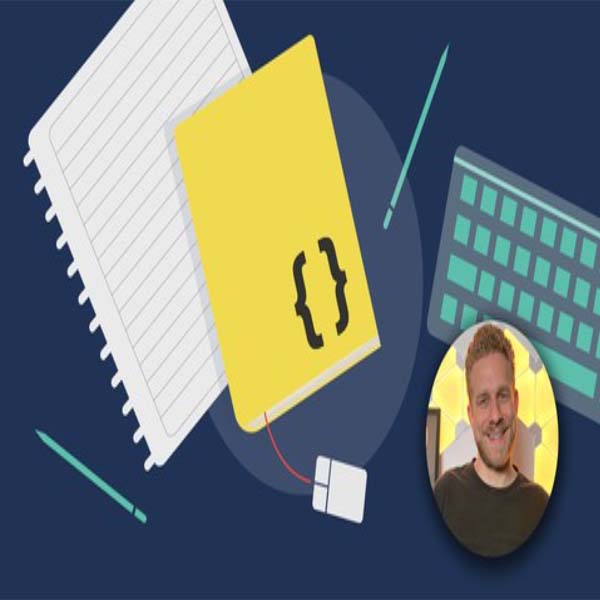
About Course
Learn JavaScript from the ground up with this comprehensive and in-depth JavaScript course. This free course covers everything from the core basics to advanced concepts, including JavaScript syntax, variables, functions, arrays, objects, control structures, DOM manipulation, events, classes, object-oriented programming, asynchronous programming, Http requests, tooling, optimizations, browser support, libraries, frameworks, Node.js, security, performance optimizations, and automated testing.
This course is perfect for beginners with no prior programming experience, as well as experienced JavaScript developers looking to enhance their skills. It is packed with examples, demos, projects, assignments, quizzes, and videos to help you learn effectively.
This course is completely free and available on Theetay, a website that offers top-rated online courses from various platforms including Udemy, Udacity, Coursera, MasterClass, NearPeer, and more.
**Key Features:**
* **Modern JavaScript Syntax:** Learn the latest JavaScript syntax from the start.
* **Comprehensive Coverage:** Covers all aspects of JavaScript, from basic concepts to advanced topics.
* **Hands-on Learning:** Includes practical examples, projects, and assignments.
* **Expert Instruction:** Taught by an experienced JavaScript developer and teacher.
* **Free Access:** Completely free to access on Theetay.
**Course Prerequisites:**
* No prior JavaScript knowledge required.
* No prior programming experience required.
* Basic HTML and CSS knowledge is recommended but not required.
**Get started today and become a JavaScript expert!**
What Will You Learn?
- Learn JavaScript from scratch and in great detail - from beginner to advanced
- All core features and concepts you need to know in modern JavaScript development
- Everything you need to become a JavaScript expert and apply for JavaScript jobs
- Project-driven learning with plenty of examples
- All about variables, functions, objects and arrays
- Object-oriented programming
- Deep dives into prototypes, JavaScript engines & how it works behind the scenes
- Manipulating web pages (= the DOM) with JavaScript
- Event handling, asynchronous coding and Http requests
- Meta-programming, performance optimization, memory leak busting
- Testing, security and deployment
- And so much more!
Course Content
Introduction
-
A Message from the Professor
-
Introduction
01:50 -
What is JavaScript_
03:46 -
JavaScript in Action_
09:08 -
How JavaScript Is Executed
03:14 -
Dynamic vs Weakly Typed Languages
03:28 -
JavaScript Executes In A Hosted Environment
04:40 -
Course Outline – What’s In This Course_
06:00 -
How To Get The Most Out Of This Course
02:36 -
JavaScript vs Java
04:02 -
A Brief History Of JavaScript
06:03 -
Setting Up a Development Environment
11:12
Basics_ Variables, Data Types, Operators & Functions
-
Module Introduction
01:17 -
Setting Up the Project
04:25 -
Adding JavaScript to the Website
06:42 -
Introducing Variables & Constants
05:17 -
Declaring & Defining Variables
07:10 -
Working with Variables & Operators
06:17 -
Understanding the Starting Code
01:21 -
Data Types_ Numbers & Strings (Text)
06:01 -
Using Constants
05:11 -
More on Strings
15:51 -
Introducing Functions
05:50 -
Adding A Custom Function
11:22 -
Returning Values
04:31 -
The (Un)Importance of Code Order
04:34 -
An Introduction to Global & Local Scope
05:31 -
More about the _return_ Statement
02:24 -
Executing Functions _Indirectly_
11:10 -
Converting Data Types
06:14 -
Splitting Code into Functions
05:49 -
Connecting all Buttons to Functions
07:44 -
Working with Code Comments
04:09 -
More Operators_
06:39 -
More Core Data Types_
04:31 -
Using Arrays
08:53 -
Creating Objects
06:02 -
Accessing Object Data
02:51 -
Adding a Re-Usable Function That Uses Objects
05:24 -
undefined
06:20 -
The _typeof_ Operator
03:12 -
Importing Scripts Correctly with _defer_ & _async_
14:37 -
Wrap Up
02:13
Efficient Development & Debugging
-
Module Introduction
01:28 -
Efficient Development & Debugging – An Overview
03:18 -
Configuring the IDE Look & Feel
02:25 -
Using Shortcuts
04:12 -
Working with Auto-Completion & IDE Hints
04:34 -
Installing IDE Extensions
02:04 -
Tweaking Editor Settings
02:15 -
Utilizing Different IDE Views
01:42 -
Finding Help & Working with MDN
05:53 -
How to _google_ Correctly
01:45 -
Debugging JavaScript – An Overview
03:17 -
An Error Message_ No Reason To Panic_
04:46 -
Using console.log() to look _into the Code_
03:49 -
Next-Level Debugging with the Chrome Devtools & Breakpoints
08:20 -
Testing Code Changes Directly in the Devtools
02:05 -
Debugging Code directly Inside VS Code
04:55 -
Wrap Up
01:22
Working with Control Structures (if Statements, Loops, Error Handling)
-
Module Introduction
02:26 -
Introducing _if_ Statements & Boolean (Comparison) Operators
09:26 -
Using _if_ Statements
07:23 -
Working with _if_
05:10 -
Beware When Comparing Objects & Arrays for Equality_
04:06 -
The Logical AND and OR Operators
09:10 -
Understanding Operator Precedence
07:20 -
Beyond true_ false_ _Truthy_ and _Falsy_ Values
07:30 -
Setting Up a Bigger Example Project (The _Monster Killer_)
02:59 -
Adding an _Attack_ Function
07:57 -
Using _if_ Statements for Checking the Win-Condition
09:17 -
Adding More _if_ Statements & A _Strong Attack_ Functionality
07:41 -
Time for a _Heal Player_ Functionality_
10:15 -
Controlling the Conditional Bonus Life (Without Boolean Operators_)
06:00 -
Adding a _Reset Game_ Functionality
06:00 -
Validating User Input
06:17 -
Utilizing Global Constants as Identifiers in Conditional Code
03:20 -
Adding a Conditional Battle Log
16:37 -
Introducing the Ternary Operator
07:31 -
A Bit of Theory_ Statements vs Expressions
01:40 -
Logical Operator _Tricks_ & Shorthands
12:58 -
Working with the _switch-case_ Statement
07:10 -
Introducing Loops
06:40 -
The _for_ Loop
07:38 -
The _for-of_ Loop
05:17 -
The _for-in_ Loop
06:49 -
The _while_ & _do-while_ Loops
08:00 -
Controlling Loops with _break_
08:11 -
Controlling Iterations with _continue_
02:21 -
More Control with Labeled Statements
06:26 -
Error Handling with _try-catch_ – An Introduction
02:25 -
Throwing Custom Errors
05:17 -
Working with _try-catch_ to Catch & Handle Errors
08:14 -
Wrap Up
03:21
Behind the Scenes & The (Weird) Past (ES3, ES5) & Present (ES6+) of JavaScript
-
Module Introduction
01:43 -
ES5 vs ES6+ (_Next Gen JS_) – Evolution of JavaScript
08:14 -
var vs let & const – Introducing _Block Scope_
14:32 -
Understanding _Hoisting_
04:07 -
Strict Mode & Writing Good Code
05:46 -
How Code is Parsed & Compiled
08:16 -
Inside the JavaScript Engine – How the Code Executes
15:59 -
Primitive vs Reference Values
19:24 -
Garbage Collection & Memory Management
12:00 -
Wrap Up
01:55
More on Functions
-
Module Introduction
00:31 -
Recapping Functions Knowledge – What We Know Thus Far
01:52 -
Functions vs Methods
05:46 -
Functions are Objects_
02:47 -
Function Expressions_ Storing Functions in Variables
05:12 -
Function Expressions vs Function Declarations
02:47 -
Anonymous Functions
05:54 -
Working on the Project_ Adding User Choices to the Game
07:44 -
Implementing the Core Game Logic
07:20 -
Introducing Arrow Functions
08:41 -
Outputting Messages to the User
03:54 -
Default Arguments in Functions
10:45 -
Introducing Rest Parameters (_Rest Operator_)
08:57 -
Creating Functions Inside of Functions
03:04 -
Understanding Callback Functions
06:09 -
Working with _bind()_
08:39 -
Adding bind() to the Calculator Project
03:47 -
call() and apply()
01:18 -
Wrap Up
02:10
Working with the DOM (Browser HTML Code) in JavaScript
-
Module Introduction
01:48 -
What’s the _DOM__
06:00 -
Document and Window Object
06:20 -
Understanding the DOM and how it’s created
07:07 -
Nodes & Elements – Querying the DOM Overview
05:55 -
Selecting Elements in the DOM
09:54 -
Exploring and Changing DOM Properties
07:38 -
Attributes vs Properties
08:58 -
Selecting Multiple Elements & Summary
05:13 -
Traversing the DOM – Overview
06:22 -
Traversing Child Nodes
09:15 -
Using parentNode & parentElement
05:01 -
Selecting Sibling Elements
04:06 -
DOM Traversal vs Query Methods
04:35 -
Styling DOM Elements
12:18 -
Creating Elements with JS – Overview
02:42 -
Adding Elements via HTML in Code
07:42 -
Adding Elements via createElement()
05:42 -
Inserting DOM Elements
08:15 -
Cloning DOM Nodes
01:45 -
Live Node Lists vs Static Node Lists
04:55 -
Removing Elements
01:56 -
Insertion & Removal Method Summary
02:38 -
Setting Up the Practice Project
02:16 -
Selecting the Modal and _Add_ Button
08:58 -
Opening a Modal by Changing CSS Classes
05:01 -
Controlling the Backdrop
08:04 -
Fetching and Validating User Input
08:06 -
Creating a Movie in JavaScript & Clearing the Input
04:01 -
Rendering Movie Items on the Screen
08:24 -
Deleting Movie Elements
09:12 -
Showing & Hiding the _Are you sure__ Dialog
07:08 -
Starting with the Confirmation Logic
04:29 -
Finishing the App
11:45 -
Wrap Up
01:55
More on Arrays & Iterables
-
Module Introduction
01:08 -
What are _Iterables_ and _Array-like Objects__
02:11 -
Creating Arrays
08:56 -
Which Data Can You Store In Arrays_
03:47 -
push()
06:59 -
The splice() Method
05:38 -
Selecting Ranges & Creating Copies with slice()
06:06 -
Adding Arrays to Arrays with concat()
02:23 -
Retrieving Indexes with indexOf() _& lastIndexOf()
03:47 -
Finding Stuff_ find() and findIndex()
05:20 -
Is it Included_
01:20 -
Alternative to for Loops_ The forEach() Method
04:25 -
Transforming Data with map()
02:38 -
sort()ing and reverse()ing
04:16 -
Filtering Arrays with filter()
02:35 -
Where Arrow Functions Shine_
01:31 -
The Important reduce() Method
07:33 -
Arrays & Strings – split() and join()
04:21 -
The Spread Operator (…)
10:31 -
Understanding Array Destructuring
04:25 -
Maps & Sets – Overview
04:16 -
Working with Sets
07:21 -
Working with Maps
09:30 -
Maps vs Objects
03:41 -
Understanding WeakSet
04:50 -
Understanding WeakMap
02:51 -
Wrap Up
01:25
More on Objects
-
Module Introduction
01:38 -
What’s an Object_
05:54 -
Objects – Recap
02:42 -
Adding
06:46 -
Special Key Names & Square Bracket Property Access
08:36 -
Property Types & Property Order
03:55 -
Dynamic Property Access & Setting Properties Dynamically
04:11 -
Demo App & Shorthand Property Syntax
09:22 -
Rendering Elements based on Objects
05:36 -
for-in Loops & Outputting Dynamic Properties
05:24 -
Adding the Filter Functionality
05:38 -
Understanding _Chaining_ (Property & Method Chaining)
01:51 -
The Object Spread Operator (…)
05:54 -
Understanding Object.assign()
02:08 -
Object Destructuring
06:13 -
Checking for Property Existance
02:42 -
Introducing _this_
05:52 -
The Method Shorthand Syntax
01:07 -
The _this_ Keyword And Its Strange Behavior
05:41 -
call() and apply()
03:22 -
What the Browser (Sometimes) Does to _this_
02:33 -
_this_ and Arrow Functions
10:36 -
Getters & Setters
07:05 -
Wrap Up
01:35
Classes & Object-oriented Programming (OOP)
-
Module Introduction
01:55 -
What is _Object-oriented Programming_ (OOP)_
03:17 -
Getting Started with OOP Code
12:10 -
Defining & Using a First Class
07:17 -
Working with Constructor Methods
04:51 -
Fields vs Properties
02:19 -
Using & _Connecting_ Multiple Classes
09:06 -
Binding Class Methods & Working with _this_
04:57 -
Adding a Cart and Shop Class
04:37 -
Communicating Can Be Challenging_
03:54 -
Static Methods & Properties
07:51 -
First Summary & Classes vs Object Literals
04:06 -
Getters & Setters
05:43 -
Introducing Inheritance
02:34 -
Implementing Inheritance
11:50 -
Using Inheritance Everywhere
06:51 -
Overriding Methods and the super() Constructor
06:00 -
super() Constructor Execution
06:46 -
Different Ways of Adding Methods
05:51 -
Private Properties
07:24 -
The _instanceof_ Operator
04:30 -
Built-in Classes
01:09 -
Understanding Object Descriptors
07:35 -
Wrap Up
01:51
Deep Dive_ Constructor Functions & Prototypes
-
Module Introduction
01:34 -
Introducing Constructor Functions
04:02 -
Constructor Functions vs Classes & Understanding _new_
04:17 -
Introducing Prototypes
16:47 -
Working with Prototypes
05:19 -
The Prototype Chain and the Global _Object_
08:26 -
Classes & Prototypes
05:24 -
Methods in Classes & In Constructors
10:16 -
Built-in Prototypes in JavaScript
02:12 -
Setting & Getting Prototypes
10:58 -
Wrap Up
02:49
Practice_ OOP & Classes
-
Module Introduction
01:38 -
First Project Steps & Planning
04:46 -
Creating Project Lists & Parsing Element Data
04:08 -
Starting with the _Switch Project_ Logic
09:59 -
Passing Method References Around
07:02 -
Moving DOM Elements
11:49 -
Adding a Tooltip
08:58 -
Adding Inheritance
06:13 -
Wrap Up
00:57
Back to the DOM & More Browser APIs
-
Module Introduction
02:31 -
Using _dataset_ (data-_ Attributes)
06:51 -
Getting Element Box Dimensions
05:54 -
Working with Element Sizes & Positions
04:56 -
The DOM & Prototypes
02:21 -
Positioning the Tooltip
10:57 -
Handling Scrolling
05:37 -
Working with _template_ Tags
05:14 -
Loading Scripts Dynamically
07:35 -
Setting Timers & Intervals
07:37 -
The _location_ and _history_ Objects
04:20 -
The _navigator_ Object
04:50 -
Working with Dates
03:17 -
The _Error_ Object & Constructor Function
03:21 -
Wrap Up
00:43
Working with Events
-
Module Introduction
01:31 -
Introduction to Events in JavaScript
06:18 -
Different Ways of Listening to Events
06:59 -
Removing Event Listeners
05:14 -
The _event_ Object
05:43 -
Supported Event Types
08:01 -
Working with _preventDefault()_
05:15 -
Understanding _Capturing_ & _Bubbling_ Phases
02:03 -
Event Propagation & _stopPropagation()_
07:39 -
Using Event Delegation
08:33 -
Triggering DOM Elements Programmatically
03:40 -
Event Handler Functions & _this_
02:28 -
Drag & Drop – Theory
04:59 -
Configuring Draggable Elements
06:23 -
Marking the _Drop Area_
08:52 -
Dropping & Moving Data + Elements
06:58 -
Wrap Up
01:15
Advanced Function Concepts
-
Module Introduction
00:57 -
Pure Functions & Side-Effects
06:13 -
Impure vs Pure Functions
02:00 -
Factory Functions
05:41 -
Closures
07:45 -
Closures in Practice
07:01 -
Closures & Memory Management
01:24 -
Introducing _Recursion_
07:32 -
Advanced Recursion
09:01 -
Wrap Up
01:14
More on Numbers & Strings
-
Module Introduction
00:53 -
How Numbers Work & Behave in JavaScript
07:46 -
Floating Point (Im)Precision
11:04 -
The BigInt Type
03:36 -
The Global _Number_ and _Math_ Objects
02:47 -
Example_ Generate Random Number Between Min_ Max
05:33 -
Exploring String Methods
01:43 -
Tagged Templates
10:29 -
Introducing Regular Expressions (_RegEx_)
04:31 -
More on Regular Expressions
07:25 -
Wrap Up
01:20
Async JavaScript_ Promises & Callbacks
-
Module Introduction
01:12 -
Understanding Synchronous Code Execution (_Sync Code_)
02:51 -
Understanding Asynchronous Code Execution (_Async Code_)
05:44 -
Blocking Code & The _Event Loop_
10:30 -
Sync + Async Code – The Execution Order
04:03 -
Multiple Callbacks & setTimeout(0)
03:20 -
Getting Started with Promises
08:25 -
Chaining Multiple Promises
05:53 -
Promise Error Handling
07:46 -
Async_ await
09:11 -
Async_ await & Error Handling
03:07 -
Async_ await vs _Raw Promises_
04:56 -
Promise.all()
07:59 -
Wrap Up
01:27
Working with Http Requests
-
Module Introduction
01:07 -
What & Why
05:03 -
More Background about Http
05:24 -
Getting Started with Http
03:35 -
Sending a GET Request
03:46 -
JSON Data & Parsing Data
09:14 -
Promisifying Http Requests (with XMLHttpRequest)
03:49 -
Sending Data with a POST Request
04:55 -
Triggering Requests via the UI
03:13 -
Sending a DELETE Request
04:56 -
Handling Errors
05:03 -
Using the fetch() API
07:11 -
POSTing Data with the fetch() API
02:38 -
Adding Request Headers
03:01 -
fetch() & Error Handling
07:12 -
XMLHttpRequest vs fetch()
01:42 -
Working with FormData
06:58 -
Wrap Up
01:17
Working with JavaScript Libraries
-
Module Introduction
00:59 -
What & Why
02:55 -
Adding Libraries (Example_ lodash)
09:10 -
Example_ jQuery
02:30 -
Discovering Libraries
03:25 -
Axios Library & Http Requests
10:46 -
Third-Party Library Considerations
04:54 -
Wrap Up
00:59
Modular JavaScript (Working with Modules)
-
Module Introduction
00:56 -
Splitting Code in a Sub-optimal Way
07:12 -
A First Step Towards JavaScript Modules
03:25 -
We Need a Development Server_
05:58 -
First import _ export Work
03:41 -
Switching All Files To Use Modules
04:26 -
More Named Export Syntax Variations
06:12 -
Working With Default Exports
03:35 -
Dynamic Imports & Code Splitting
05:24 -
When Does Module Code Execute_
02:06 -
Module Scope & globalThis
06:18 -
Wrap Up
01:37
JavaScript Tooling & Workflows
-
Module Introduction
03:23 -
Project Limitations & Why We Need Tools
08:11 -
Workflow Overview
02:42 -
Setting Up a npm Project
03:46 -
Working with npm Packages
03:39 -
Linting with ESLint
08:38 -
Bundling with Webpack
15:13 -
Development Mode & Fixing _Lazy Loading_
04:00 -
Using webpack-dev-server
03:14 -
Generating Sourcemaps
03:05 -
Building For Production
03:45 -
Final Optimizations
06:35 -
Using Third Party Packages with npm & Webpack
00:06 -
Wrap Up
01:39
Utilizing Browser Storage
-
Module Introduction
01:06 -
Browser Storage Options
07:21 -
localStorage & sessionStorage
10:43 -
Getting Started with Cookies
06:06 -
Working with Cookies
08:10 -
Getting Started with IndexedDB
08:55 -
Working with IndexedDB
04:06 -
Wrap Up
00:48
JavaScript & Browser Support
-
Module Introduction
01:29 -
What Is _Browser Support_ About_
07:36 -
Determining Browser Support For A JavaScript Feature
08:20 -
Determining Required Support
03:29 -
Solution_ Feature Detection + Fallback Code
09:55 -
Solution_ Using Polyfills
03:18 -
Solution_ Transpiling Code
12:02 -
Improvement_ Automatically Detect + Add Polyfills
11:02 -
What about Support Outside of Browsers_
00:58 -
Browser Support Outside of JavaScript Files
02:36 -
Wrap Up
01:18
Time to Practice_ Share My Place App
-
Module Introduction
00:53 -
Setting Up the Project
03:05 -
Getting DOM Access
04:33 -
Getting the User Location
06:27 -
Adding Feedback (Showing a Modal)
11:16 -
Hiding the Modal
02:38 -
Rendering a Map with Google Maps
14:16 -
Finding an Address & Getting the Coordinates
08:22 -
Converting User Input to Coordinates
03:35 -
Creating a _Share Place_ Link
08:31 -
Copying the Link to the Clipboard
03:36 -
Rendering the _Shared Place_ Screen
06:22
Working with JavaScript Frameworks
-
Module Introduction
01:06 -
What and Why_
07:35 -
The Idea Behind React.js
02:46 -
Analysing a React Project
17:38 -
Wrap Up
01:35
Meta-Programming_ Symbols, Iterators, Generators, Reflect API & Proxy API
-
Module Introduction
01:51 -
Understanding Symbols
08:27 -
Well-known Symbols
05:15 -
Understanding Iterators
05:32 -
Generators & Iterable Objects
11:02 -
Generators Summary & Built-in Iterables Examples
03:22 -
The Reflect API
07:10 -
The Proxy API and a First _Trap_
09:04 -
Working with Proxy Traps
02:57 -
Wrap Up
01:37
Node.js_ An Introduction
-
Module Introduction
01:42 -
JavaScript is a Hosted Language
02:21 -
Installation & Basics
06:17 -
Understanding Modules & File Access
04:39 -
Working with Incoming Http Requests
05:46 -
Sending Responses (HTML Data)
04:01 -
Parsing Incoming Data
10:53 -
Introducing & Installing Express.js
02:48 -
Express.js_ The Basics
06:34 -
Extracting Data
04:23 -
Rendering Server-side HTML with Templates & EJS
06:52 -
Enhancing Our Project
03:44 -
Adding Basic REST Routes
12:09 -
Understanding CORS (Cross Origin Resource Sharing)
05:13 -
Sending the Location ID to the Frontend
02:16 -
Adding the GET Location Route
07:16 -
Introducing MongoDB (Database)
14:44 -
Wrap Up
01:50
Security
-
Module Introduction
01:35 -
Security Hole Overview & Exposing Data in your Code
06:45 -
Cross-Site Scripting Attacks (XSS)
14:39 -
Third-Party Libraries & XSS
05:17 -
CSRF Attacks (Cross Site Request Forgery)
04:16 -
CORS (Cross Origin Resource Sharing)
02:53 -
Wrap Up
01:31
Deploying JavaScript Code
-
Module Introduction
01:11 -
Deployment Steps
07:29 -
Example_ Static Host Deployment (no Server-side Code)
09:43 -
Example_ Dynamic Page Deployment (with Server-side Code)
12:45
Performance & Optimizations
-
Module Introduction
01:49 -
What is _Performance Optimization_ About_
06:16 -
Optimization Potentials
06:48 -
Measuring Performance
03:42 -
Diving Into The Browser Devtools (for Performance Measuring)
15:30 -
Further Resources
02:13 -
Preparing The Testing Setup
04:03 -
Optimizing Startup Time & Code Usage _ Coverage
11:51 -
Updating The DOM Correctly
09:53 -
Updating Lists Correctly
08:11 -
Optimizing The Small Things
03:58 -
Micro-Optimizations (Think Twice_)
08:49 -
Finding & Fixing Memory Leaks
09:43 -
Wrap Up
03:26
Introduction to Testing
-
Module Introduction
00:49 -
What Is Testing_ Why Does It Matter_
07:09 -
Testing Setup
04:10 -
Writing & Running Unit Tests
11:15 -
Writing & Running Integration Tests
06:03 -
Writing & Running e2e Tests
10:16 -
Dealing with Async Code
10:20 -
Working with Mocks
07:51
Bonus_ Programming Paradigms (Procedural vs Object Oriented vs Functional)
-
Module Introduction
00:48 -
What are Programming Paradigms_
03:01 -
Procedural Programming in Practice
08:53 -
Object Oriented Programming in Practice
13:20 -
Functional Programming in Practice
13:52 -
Wrap Up
03:26
Bonus_ Data Structures & Algorithms Introduction
-
Module Introduction
02:02 -
What are _Data Structures_ & _Algorithms__
04:22 -
A First Example
10:22 -
Solving the Same Problem Differently
07:26 -
Performance & The _Big O_ Notation
11:44 -
More Time Complexities & Comparing Algorithms
03:36 -
More on Big O
05:23 -
More Examples
10:15 -
Diving into Data Structures & Time Complexities
12:07 -
Where to Learn More & Wrap Up
03:23
Bonus_ TypeScript Introduction
-
Module Introduction
01:11 -
What is TypeScript and Why would you use it_
04:58 -
Working with Types
10:05 -
Core Types & Diving Deeper
17:26 -
Object Types
09:22 -
Advanced Types (Literal Types
09:20 -
Classes & Interfaces
12:02 -
Generic Types
05:02 -
Configuring the TypeScript Compiler
05:11
Bonus_ Web Components
-
Module Introduction
02:01 -
Web Components in Action
06:30 -
What are Web Components_
03:22 -
Why Web Components_
02:44 -
Getting Started_
04:56 -
Web Component Browser Support
02:44 -
Our Development Setup
03:53 -
A First Custom Element
08:18 -
Interacting with the Surrounding DOM
05:04 -
Understanding the Custom Element Lifecycle
02:51 -
Using _connectedCallback_ for DOM Access
02:05 -
Listening to Events Inside the Component
09:13 -
Using Attributes on Custom Elements
04:45 -
Styling our Elements
04:08 -
Working with the _Shadow DOM_
05:00 -
Adding an HTML Template
05:41 -
Using Slots
01:50 -
Defining the Template in JavaScript
03:21 -
Using Style Tags in the Shadow DOM
02:40 -
Extending Built-in Elements
07:33 -
The Next Steps
00:49 -
Understanding Shadow DOM Projection
02:26 -
Styling _slot_ Content Outside of the Shadow DOM
03:45 -
Styling _slot_ Content Inside of the Shadow DOM
03:10 -
Styling the Host Component
04:42 -
Conditional Host Styling
02:49 -
Styling with the Host Content in Mind
02:28 -
Smart Dynamic Styling with CSS Variables
06:20 -
Cleaning Up the Overall Styling
03:22 -
Observing Attribute Changes
06:17 -
Adjusting the Component Behavior Upon Attribute Changes
02:34 -
Using _disconnectedCallback_
06:10 -
Adding a render() Method
06:13 -
The Next Steps
00:52 -
Creating the Basic Modal Component
06:33 -
Adding the Modal Container
02:30 -
Styling the Modal Elements
05:35 -
Adding Some General App Logic
03:49 -
Opening the Modal via CSS
05:23 -
Public Methods & Properties
06:37 -
Understanding Named Slots
05:45 -
Listening to Slot Content Changes
05:10 -
Closing the Modal
06:57 -
Dispatching Custom Events
03:40 -
Configuring Custom Events
04:53 -
Finishing it up_
04:51
Roundup & Next Steps
-
Congratulations_
06:09
Earn a certificate
Add this certificate to your resume to demonstrate your skills & increase your chances of getting noticed.
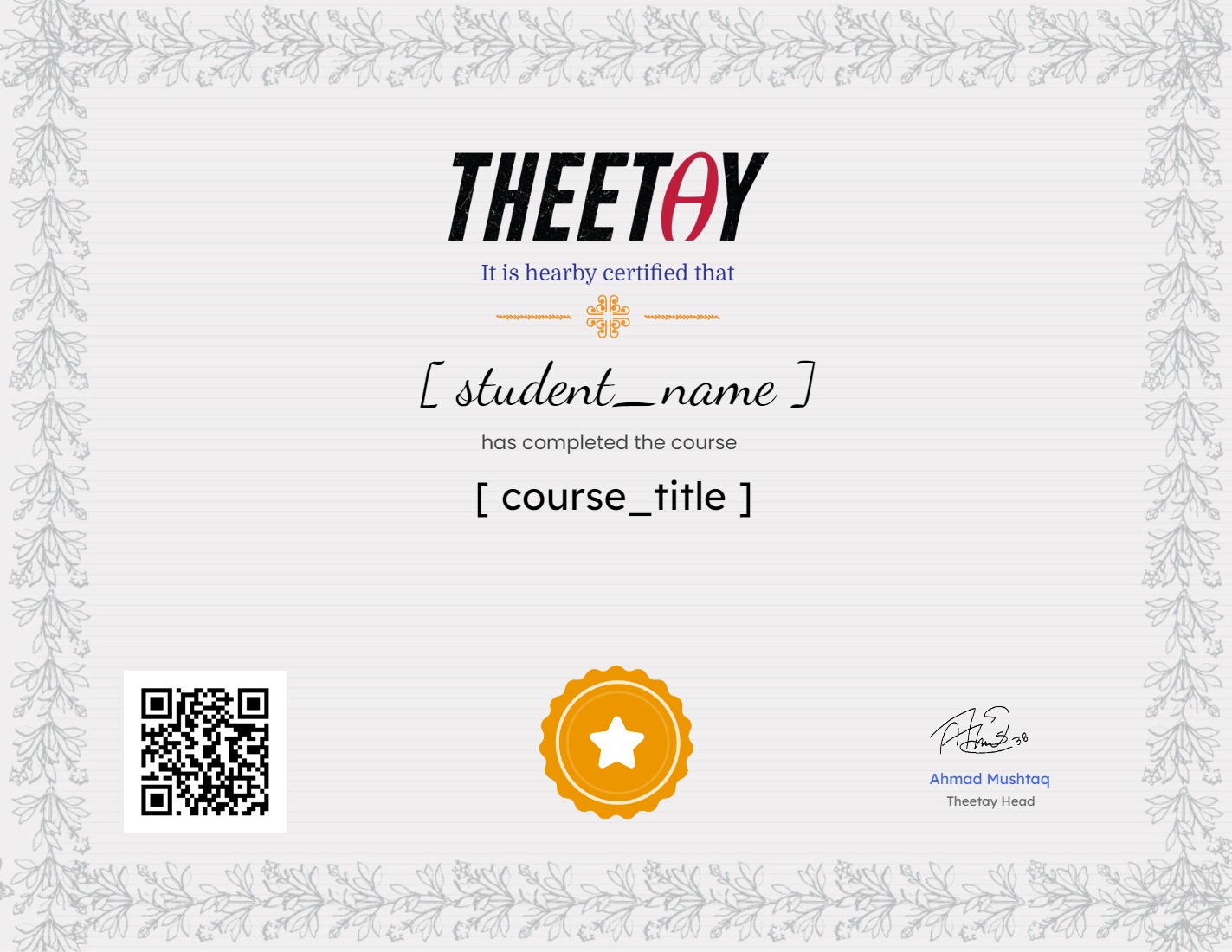