The Web Developer Bootcamp 2023
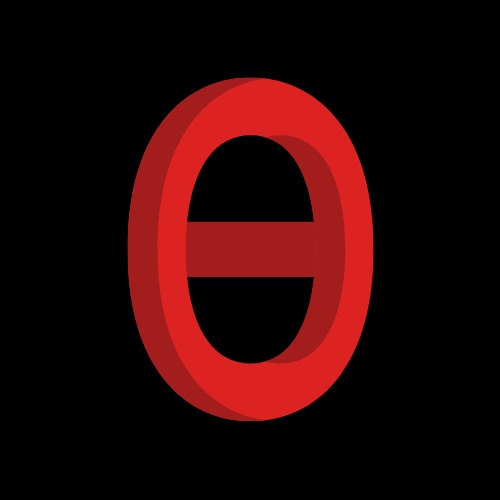
About Course
Learn how to become a web developer with this comprehensive course from Udemy. This free course is designed for complete beginners and covers all the essential web development skills, including HTML5, CSS3, JavaScript, ReactJS, NodeJS, ExpressJS, MongoDB, and more. You’ll build 13+ projects, including a gigantic production application called YelpCamp. This course is constantly updated with new content, projects, and modules. Start your journey to becoming a web developer today!
This course covers the following topics:
- HTML5
- CSS3
- Flexbox
- Responsive Design
- JavaScript (all 2022 modern syntax, ES6, ES2018, etc.)
- Asynchronous JavaScript – Promises, async/await, etc.
- AJAX and single page apps
- Bootstrap 4 and 5
- ReactJS
- SemanticUI
- Bulma CSS Framework
- DOM Manipulation
- Unix(Command Line) Commands
- NodeJS
- NPM
- ExpressJS
- Templating
- REST
- SQL vs. NoSQL databases
- MongoDB
- Database Associations
- Schema Design
- Mongoose
- Authentication From Scratch
- Cookies & Sessions
- Authorization
- Common Security Issues – SQL Injection, XSS, etc.
- Developer Best Practices
- Deploying Apps
- Cloud Databases
- Image Upload and Storage
- Maps and Geocoding
This course is taught by a professional bootcamp instructor with a track record of success. This free course is available on Theetay, which offers a wide selection of free courses from platforms like Udemy, Udacity, Coursera, MasterClass, NearPeer, and more. Start learning today!
What Will You Learn?
- The ins and outs of HTML5, CSS3, and Modern JavaScript for 2021
- Make REAL web applications using cutting-edge technologies
- Create responsive, accessible, and beautiful layouts
- Recognize and prevent common security exploits like SQL-Injection & XSS
- Continue to learn and grow as a developer, long after the course ends
- Create a blog application from scratch using Node, Express, and MongoDB.
- Create a complicated yelp-like application from scratch
- Deploy your applications and work with cloud databases
- Create static HTML and CSS portfolio sites and landing pages
- Think like a developer. Become an expert at Googling code questions!
- Create complex HTML forms with validations
- Implement full authentication from scratch!
- Use CSS Frameworks including Bootstrap 5, Semantic UI, and Bulma
- Implement responsive navbars on websites
- Use JavaScript variables, conditionals, loops, functions, arrays, and objects
- Write Javascript functions, and understand scope and higher order functions
- Master the "weird" parts of JavaScript
- Create full-stack web applications from scratch
- Manipulate the DOM with vanilla JS
- Write JavaScript based browser games
- Use Postman to monitor and test APIs
- Use NodeJS to write server-side JavaScript
- Write complex web apps with multiple models and data associations
- Write a REAL application using everything in the course
- Use Express and MongoDB to create full-stack JS applications
- Use common JS data structures like Arrays and Objects
- Master the command line interface
- Use NPM to install all sorts of useful packages
- Understand the ins and outs of HTTP requests
- Create your own Node modules
- Make a beautiful, responsive photographer's portfolio page
- Create a beautiful, responsive landing page for a startup
- Implement user authentication
- Create a beautiful animated todo list application
- Create single page applications with AJAX
Course Content
01 – Course Orientation
-
001 Welcome To The Course!.mp4
00:00 -
002 Joining The Community Chat & Groups.html
00:00 -
003 Curriculum Walkthrough.mp4
00:00 -
004 When Was The Course Last Updated.mp4
00:00 -
005 Course Change Log.html
00:00 -
006 Will I Get A Job.mp4
00:00 -
007 Accessing Course Code & Slides.mp4
00:00 -
008 Tips On The Interactive Coding Exercises.mp4
00:00 -
009 Course Coding Exercise Solutions.html
00:00 -
010 Migrating From The Old Version Of This Course.mp4
00:00 -
external-links.txt
00:00 -
Section Quiz
02 – An Introduction to Web Development
-
001 What Matters In This Section.mp4
00:00 -
002 The Internet in 5 Minutes.mp4
00:00 -
003 Intro to the Web.mp4
00:00 -
004 The RequestResponse Cycle.mp4
00:00 -
005 Front-End and Back-End.mp4
00:00 -
006 What do HTMLCSSJS do.mp4
00:00 -
007 Setting Up Our Developer Environment.mp4
00:00 -
008 OPTIONAL VSCode Theme.mp4
00:00 -
external-links.txt
00:00 -
Section Quiz
03 – HTML The Essentials
-
001 What Matters In This Section.mp4
00:00 -
002 Introduction to HTML.mp4
00:00 -
003 Our Very First HTML Page.mp4
00:00 -
004 TIP Mozilla Developer Network.mp4
00:00 -
005 Paragraph Elements.mp4
00:00 -
006 Heading Elements.mp4
00:00 -
007 Pangolin Practice.html
00:00 -
008 Introduction to the Chrome Inspector.mp4
00:00 -
009 HTML Boilerplate.mp4
00:00 -
010 VSCode Tip Auto-format.mp4
00:00 -
011 List Elements.mp4
00:00 -
012 Favorite Movies Exercises.html
00:00 -
013 Anchor Tags.mp4
00:00 -
014 Images.mp4
00:00 -
015 Comments.mp4
00:00 -
016 Wolf Images & Links Exercise.html
00:00 -
external-links.txt
00:00 -
Section Quiz
04 – HTML Next Steps & Semantics
-
001 What Matters In This Section.mp4
00:00 -
002 What Exactly Is HTML5.mp4
00:00 -
003 Block vs. Inline Elements – Divs and Spans.mp4
00:00 -
004 An Odd Assortment of Elements HR, BR, Sup, & Sub.mp4
00:00 -
005 Entity Codes.mp4
00:00 -
006 Snowman Logo Exercise.html
00:00 -
007 Intro to Semantic Markup.mp4
00:00 -
008 Playing With Semantic Elements.mp4
00:00 -
009 Screen Reader Demonstration.mp4
00:00 -
010 VSCode Tip Emmet.mp4
00:00 -
external-links.txt
00:00 -
Section Quiz
05 – HTML Forms & Tables
-
001 What Matters In This Section.mp4
00:00 -
002 Unit Goals.mp4
00:00 -
003 Introducing HTML Tables.mp4
00:00 -
004 Tables TR, TD, and TH Elements.mp4
00:00 -
005 Tables Thead, Tbody, and Tfoot Elements.mp4
00:00 -
006 Tables Colspan & Rowspan.mp4
00:00 -
007 Table Practice Exercise.html
00:00 -
008 The Form Element.mp4
00:00 -
009 Common Input Types.mp4
00:00 -
010 The All-Important Label.mp4
00:00 -
011 HTML Buttons.mp4
00:00 -
012 The Name Attribute.mp4
00:00 -
013 Hijacking Google & Reddit’s Search.mp4
00:00 -
014 Radio Buttons, Checkboxes, & Selects.mp4
00:00 -
015 Range & Text Area.mp4
00:00 -
016 Forms Practice Exercise.html
00:00 -
017 HTML5 Form Validations.mp4
00:00 -
018 Creating A Marathon Registration Form Intro.mp4
00:00 -
019 Creating A Marathon Registration Form Solution.mp4
00:00 -
external-links.txt
00:00 -
Section Quiz
06 – CSS The Very Basics
-
001 What Matters In This Section.mp4
00:00 -
002 What is CSS.mp4
00:00 -
003 CSS is Huge, Don’t Panic!.mp4
00:00 -
004 Including Styles Correctly.mp4
00:00 -
005 Color & Background-Color Properties.mp4
00:00 -
006 Our First CSS Exercise.html
00:00 -
007 Colors Systems RGB & Named Colors.mp4
00:00 -
008 Colors Systems Hexadecimal.mp4
00:00 -
009 Colors Quiz.html
00:00 -
010 A Reminder On Semicolons & CSS.mp4
00:00 -
011 Common Text Properties.mp4
00:00 -
012 Font Size Basics With Pixels.mp4
00:00 -
013 The Font Family Property.mp4
00:00 -
014 Hipster Logo Exercise.html
00:00 -
external-links.txt
00:00 -
Section Quiz
07 – The World of CSS Selectors
-
001 What Matters In This Section.mp4
00:00 -
002 Universal & Element Selectors.mp4
00:00 -
003 The ID Selector.mp4
00:00 -
004 The Class Selector.mp4
00:00 -
005 Basic Selectors Practice.html
00:00 -
006 The Descendant Selector.mp4
00:00 -
007 Descendant Combinator Practice.html
00:00 -
008 The Adjacent & Direct-Descendant Selectors.mp4
00:00 -
009 The Attribute Selector.mp4
00:00 -
010 Pseudo Classes.mp4
00:00 -
011 Checkerboard Exercise.html
00:00 -
012 Pseudo Elements.mp4
00:00 -
013 The CSS Cascade.mp4
00:00 -
014 WTF is Specificity.mp4
00:00 -
015 Specificity Quiz.html
00:00 -
016 TIP Chrome Dev Tools & CSS.mp4
00:00 -
017 Inline Styles & Important.mp4
00:00 -
018 CSS Inheritance.mp4
00:00 -
external-links.txt
00:00 -
Section Quiz
08 – The CSS Box Model
-
001 What Matters In This Section.mp4
00:00 -
002 Box Model Width & Height.mp4
00:00 -
003 Box Model Border & Border-Radius.mp4
00:00 -
004 Box Model Practice.html
00:00 -
005 Box Model Padding.mp4
00:00 -
006 Box Model Margin.mp4
00:00 -
007 The Display Property.mp4
00:00 -
008 CSS Units Revisited.mp4
00:00 -
009 CSS Units ems.mp4
00:00 -
010 CSS Units rems.mp4
00:00 -
external-links.txt
00:00 -
Section Quiz
09 – Other Assorted Useful CSS Properties
-
001 What Matters In This Section.mp4
00:00 -
002 Opacity & The Alpha Channel.mp4
00:00 -
003 The Position Property.mp4
00:00 -
004 CSS Transitions (yay!).mp4
00:00 -
005 The Power of CSS Transforms.mp4
00:00 -
006 Fancy Button Hover Effect CodeAlong.mp4
00:00 -
007 The Truth About Background.mp4
00:00 -
008 Google Fonts is Amazing.mp4
00:00 -
009 IMPORTANT REMINDER How To Find And Download Course Code.html
00:00 -
010 Photo Blog CodeAlong Pt. 1.mp4
00:00 -
011 Photo Blog CodeAlong Pt. 2.mp4
00:00 -
external-links.txt
00:00 -
Section Quiz
10 – Responsive CSS & Flexbox
-
001 What Matters In This Section.mp4
00:00 -
002 What on Earth Is Flexbox.mp4
00:00 -
003 Flex-Direction.mp4
00:00 -
004 Justify-Content.mp4
00:00 -
005 Flex-Wrap.mp4
00:00 -
006 Align-Items.mp4
00:00 -
007 Align-Content & Align-Self.mp4
00:00 -
008 Flex-Basis, Grow, & Shrink.mp4
00:00 -
009 Flex Shorthand.mp4
00:00 -
010 Responsive Design & Media Queries Intro.mp4
00:00 -
011 The Power of Media Queries.mp4
00:00 -
012 NOTE Fixing The Code Order In The Next Lecture.html
00:00 -
013 Building a Responsive Nav.mp4
00:00 -
external-links.txt
00:00 -
Section Quiz
11 – Pricing Panel Project
-
001 Pricing Panel CodeAlong Pt. 1.mp4
00:00 -
002 Pricing Panel CodeAlong Pt. 2.mp4
00:00 -
003 Pricing Panel CodeAlong Pt. 3.mp4
00:00 -
004 Pricing Panel CodeAlong Pt. 4.mp4
00:00 -
005 Pricing Panel CodeAlong Pt. 5.mp4
00:00 -
006 Pricing Panel CodeAlong Pt. 6.mp4
00:00 -
Section Quiz
12 – CSS Frameworks Bootstrap
-
001 What Matters In This Section.mp4
00:00 -
002 WTF Is Bootstrap.mp4
00:00 -
003 Including Bootstrap & Containers.mp4
00:00 -
004 Bootstrap Buttons.mp4
00:00 -
005 Bootstrap Typography & Utilities.mp4
00:00 -
006 Badges, Alerts, & Button Groups.mp4
00:00 -
007 Bootstrap Basics Practice.html
00:00 -
008 Intro to the Bootstrap Grid.mp4
00:00 -
009 Bootstrap Grid Practice.html
00:00 -
010 Responsive Bootstrap Grids.mp4
00:00 -
011 Useful Grid Utilities.mp4
00:00 -
012 Bootstrap & Forms.mp4
00:00 -
013 Bootstrap Navbars.mp4
00:00 -
014 Bootstrap Icons!.mp4
00:00 -
015 Other Bootstrap Utilities.mp4
00:00 -
016 A Mixed Bag of Other Bootstrap Stuff.mp4
00:00 -
external-links.txt
00:00 -
Section Quiz
13 – OPTIONAL Museum Of Candy Project
-
001 Watch This First! (it’s short).mp4
00:00 -
002 Museum of Candy Project Part 1.mp4
00:00 -
003 NOTE ABOUT THE NEXT VIDEO!.html
00:00 -
004 Museum of Candy Project Part 2.mp4
00:00 -
005 Museum of Candy Project Part 3.mp4
00:00 -
006 Museum of Candy Project Part 4.mp4
00:00 -
007 Museum of Candy Project Part 5.mp4
00:00 -
Section Quiz
14 – JavaScript Basics!
-
001 What Matters In This Section.mp4
00:00 -
002 Why JavaScript is Awesome.mp4
00:00 -
003 Primitives & The Console.mp4
00:00 -
004 JavaScript Numbers.mp4
00:00 -
005 WTF is NaN.mp4
00:00 -
006 Quick Numbers Quiz.mp4
00:00 -
007 Variables & Let.mp4
00:00 -
008 Our First Variables Exercise.html
00:00 -
009 Updating Variables.mp4
00:00 -
010 Increment Operator Explanation i++ vs ++i.mp4
00:00 -
011 Const & Var.mp4
00:00 -
012 Our First Constants Exercise.html
00:00 -
013 Variables Quiz.html
00:00 -
014 Booleans.mp4
00:00 -
015 Variable Naming and Conventions.mp4
00:00 -
016 Quick Variable Quiz.html
00:00 -
external-links.txt
00:00 -
Section Quiz
15 – JavaScript Strings and More
-
001 What Matters In This Section.mp4
00:00 -
002 Introducing Strings.mp4
00:00 -
003 Our First String Variables Practice.html
00:00 -
004 Indices & Length.mp4
00:00 -
005 Strings Basics Quiz.html
00:00 -
006 String Methods.mp4
00:00 -
007 String Methods Practice.html
00:00 -
008 String Methods With Arguments.mp4
00:00 -
009 More String Methods Practice.html
00:00 -
010 String Template Literals -SUPER USEFUL.mp4
00:00 -
011 Undefined & Null.mp4
00:00 -
012 Random Numbers & The Math Object.mp4
00:00 -
013 String Template Literal Exercise.html
00:00 -
external-links.txt
00:00 -
Section Quiz
16 – JavaScript Decision Making
-
001 What Matters In This Section.mp4
00:00 -
002 Decision Making With Code.mp4
00:00 -
003 Comparison Operators.mp4
00:00 -
004 Equality Triple Vs. Double Equals.mp4
00:00 -
005 Comparison Quiz!.html
00:00 -
006 Console, Alert, & Prompt.mp4
00:00 -
007 Running JavaScript From A Script!.mp4
00:00 -
008 If Statements.mp4
00:00 -
009 Our First Conditional Exercise.html
00:00 -
010 Else-If.mp4
00:00 -
011 Else.mp4
00:00 -
012 getColor Conditional Exercise.html
00:00 -
013 Nesting Conditionals.mp4
00:00 -
014 Nested Conditionals Practice.html
00:00 -
015 Truth-y & False-y Values.mp4
00:00 -
016 Logical AND.mp4
00:00 -
017 Logical AND Mystery Exercise.html
00:00 -
018 Logical OR.mp4
00:00 -
019 Logical NOT.mp4
00:00 -
020 The Switch Statement Is…A Lot.mp4
00:00 -
external-links.txt
00:00 -
Section Quiz
17 – JavaScript Arrays
-
001 What Matters In This Section.mp4
00:00 -
002 Introducing Arrays.mp4
00:00 -
003 Lotto Numbers Exercise.html
00:00 -
004 Array Random Access.mp4
00:00 -
005 Array Access Exercise.html
00:00 -
006 Push & Pop.mp4
00:00 -
007 Shift & Unshift.mp4
00:00 -
008 PushPopShiftUnshift Practice.html
00:00 -
009 Concat, indexOf, includes & reverse.mp4
00:00 -
010 Slice & Splice.mp4
00:00 -
011 Reference Types & Equality Testing.mp4
00:00 -
012 Arrays + Const.mp4
00:00 -
013 Multi-Dimensional Arrays.mp4
00:00 -
014 Nested Arrays Exercise.html
00:00 -
external-links.txt
00:00 -
Section Quiz
18 – JavaScript Object Literals
-
001 What Matters In This Section.mp4
00:00 -
002 Introducing Object Literals.mp4
00:00 -
003 Creating Object Literals.mp4
00:00 -
004 Our First Object Exercise.html
00:00 -
005 Accessing Data Out Of Objects.mp4
00:00 -
006 Object Access Exercise.html
00:00 -
007 Modifying Objects.mp4
00:00 -
008 Nesting Arrays & Objects.mp4
00:00 -
external-links.txt
00:00 -
Section Quiz
19 – Repeating Stuff With Loops
-
001 What Matters In This Section.mp4
00:00 -
002 Intro to For Loops.mp4
00:00 -
003 Our First For Loop Practice.html
00:00 -
004 More For Loops Examples.mp4
00:00 -
005 More For Loops Practice.html
00:00 -
006 The Perils Of Infinite Loops (.mp4
00:00 -
007 Looping Over Arrays.mp4
00:00 -
008 Iterating Arrays Exercise.html
00:00 -
009 Nested Loops.mp4
00:00 -
010 Another Loop The While Loop.mp4
00:00 -
011 The Break Keyword.mp4
00:00 -
012 Writing a Guessing Game.mp4
00:00 -
013 Guessing Game Explanation And Bug Fixes.mp4
00:00 -
014 The Lovely For…Of Loop.mp4
00:00 -
015 For…Of Practice.html
00:00 -
016 Iterating Over Objects.mp4
00:00 -
017 Todo List Project Intro.mp4
00:00 -
018 Todo List Project CodeAlong.mp4
00:00 -
external-links.txt
00:00 -
Section Quiz
20 – NEW Introducing Functions
-
001 What Matters In This Section.mp4
00:00 -
002 Intro to Functions.mp4
00:00 -
003 Our Very First Function.mp4
00:00 -
004 Heart Function Exercise.html
00:00 -
005 Arguments Intro.mp4
00:00 -
006 Rant Exercise.html
00:00 -
007 Functions With Multiple Arguments.mp4
00:00 -
008 Multiple Args Exercise.html
00:00 -
009 The Return Keyword.mp4
00:00 -
010 Return Value Practice.html
00:00 -
011 isShortsWeather Function.html
00:00 -
012 Last Element Exercise.html
00:00 -
013 Capitalize Exercise.html
00:00 -
014 Sum Array Exercise.html
00:00 -
015 Days Of The Week Exercise.html
00:00 -
external-links.txt
00:00 -
Section Quiz
21 – Leveling Up Our Functions
-
001 What Matters In This Section.mp4
00:00 -
002 Function Scope.mp4
00:00 -
003 Function Scope Quiz.html
00:00 -
004 Block Scope.mp4
00:00 -
005 Lexical Scope.mp4
00:00 -
006 Function Expressions.mp4
00:00 -
007 Function Expression Exercise.html
00:00 -
008 Higher Order Functions.mp4
00:00 -
009 Returning Functions.mp4
00:00 -
010 Defining Methods.mp4
00:00 -
011 Methods Exercise.html
00:00 -
012 The Mysterious Keyword ‘this’.mp4
00:00 -
013 Egg Laying Exercise.html
00:00 -
014 Using TryCatch.mp4
00:00 -
external-links.txt
00:00 -
Section Quiz
22 – Callbacks & Array Methods
-
001 What Matters In This Section.mp4
00:00 -
002 What Is This Section Even About!.mp4
00:00 -
003 The forEach Method.mp4
00:00 -
004 The map Method.mp4
00:00 -
005 Map Practice.html
00:00 -
006 Intro to Arrow Functions.mp4
00:00 -
007 Arrow Function Exercise.html
00:00 -
008 Arrow Function Implicit Returns.mp4
00:00 -
009 Arrow Functions Wrapup.mp4
00:00 -
010 setTimeout and setInterval.mp4
00:00 -
011 The filter Method.mp4
00:00 -
012 Filter Exercise.html
00:00 -
013 Some & Every Methods.mp4
00:00 -
014 SomeEvery Exercise.html
00:00 -
015 The Notorious Reduce Method.mp4
00:00 -
016 Arrow Functions & ‘this’.mp4
00:00 -
external-links.txt
00:00 -
Section Quiz
23 – Newer JavaScript Features
-
001 What Matters In This Section.mp4
00:00 -
002 Default Params.mp4
00:00 -
003 Spread in Function Calls.mp4
00:00 -
004 Spread with Array Literals.mp4
00:00 -
005 Spread with Objects.mp4
00:00 -
006 Rest Params.mp4
00:00 -
007 Destructuring Arrays.mp4
00:00 -
008 Destructuring Objects.mp4
00:00 -
009 Destructuring Params.mp4
00:00 -
external-links.txt
00:00 -
Section Quiz
24 – Introducing The World Of The DOM
-
001 What Matters In This Section.mp4
00:00 -
002 Introducing the DOM.mp4
00:00 -
003 The Document Object.mp4
00:00 -
004 getElementById.mp4
00:00 -
005 getElementById Practice.html
00:00 -
006 getElementsByTagName & className.mp4
00:00 -
007 querySelector & querySelectorAll.mp4
00:00 -
008 querySelector Practice.html
00:00 -
009 innerHTML, textContent, & innerText.mp4
00:00 -
010 Pickles Exercise.html
00:00 -
011 Attributes.mp4
00:00 -
012 Manipulating Attributes Practice.html
00:00 -
013 Changing Styles.mp4
00:00 -
014 Magical Forest Circle Exercise.html
00:00 -
015 Rainbow Text Exercise.html
00:00 -
016 ClassList.mp4
00:00 -
017 ClassList Practice.html
00:00 -
018 Traversing ParentChildSibling.mp4
00:00 -
019 Append & AppendChild.mp4
00:00 -
020 100 Button Insanity Exercise.html
00:00 -
021 removeChild & remove.mp4
00:00 -
022 Pokemon Sprites Demo.mp4
00:00 -
external-links.txt
00:00 -
Section Quiz
25 – The Missing Piece DOM Events
-
001 What Matters In This Section.mp4
00:00 -
002 Intro to Events.mp4
00:00 -
003 Inline Events.mp4
00:00 -
004 Know Thy Enemy Exercise.html
00:00 -
005 The Onclick Property.mp4
00:00 -
006 addEventListener.mp4
00:00 -
007 Click Events Exercise.html
00:00 -
008 Random Color Exercise.mp4
00:00 -
009 Events & The Keyword This.mp4
00:00 -
010 Keyboard Events & Event Objects.mp4
00:00 -
011 NEW VERSION Form Events & PreventDefault.mp4
00:00 -
012 NEW VERSION Practice With Form Events & PreventDefault.mp4
00:00 -
013 ORIGINAL VERSION Form Events & PreventDefault.mp4
00:00 -
014 Form Events Exercise.html
00:00 -
015 Input & Change Events.mp4
00:00 -
016 Input Event Practice.html
00:00 -
017 Event Bubbling.mp4
00:00 -
018 Event Delegation.mp4
00:00 -
Section Quiz
26 – Score Keeper CodeAlong
-
001 Score Keeper Pt. 1.mp4
00:00 -
002 Score Keeper Pt. 2.mp4
00:00 -
003 Score Keeper Pt. 3 With Bulma.mp4
00:00 -
004 Score Keeper Pt. 4 Refactoring.mp4
00:00 -
external-links.txt
00:00 -
Section Quiz
27 – Async JavaScript Oh Boy!
-
001 What Matters In This Section.mp4
00:00 -
002 The Call Stack.mp4
00:00 -
003 WebAPIs & Single Threaded.mp4
00:00 -
004 Callback Hell (.mp4
00:00 -
005 Demo fakeRequest Using Callbacks.mp4
00:00 -
006 Demo fakeRequest Using Promises.mp4
00:00 -
007 The Magic Of Promises.mp4
00:00 -
008 Creating Our Own Promises.mp4
00:00 -
009 The Async Keyword.mp4
00:00 -
010 The Await Keyword.mp4
00:00 -
011 Handling Errors In Async Functions.mp4
00:00 -
external-links.txt
00:00 -
Section Quiz
28 – AJAX and API’s
-
001 What Matters In This Section.mp4
00:00 -
002 Intro to AJAX.mp4
00:00 -
003 Intro to APIs.mp4
00:00 -
004 WTF is JSON.mp4
00:00 -
005 Using Hoppscotch (or Postman).mp4
00:00 -
006 HTTP Verbs.mp4
00:00 -
007 HTTP Status Codes.mp4
00:00 -
008 Understanding Query Strings.mp4
00:00 -
009 HTTP Headers.mp4
00:00 -
010 Making XHRs.mp4
00:00 -
011 Using The Fetch API.mp4
00:00 -
012 Introducing Axios.mp4
00:00 -
013 Setting Headers With Axios.mp4
00:00 -
014 TV Show Search App.mp4
00:00 -
external-links.txt
00:00 -
Section Quiz
29 – Prototypes, Classes, & OOP
-
001 What Matters In This Section.mp4
00:00 -
002 What On Earth Are Prototypes.mp4
00:00 -
003 Intro to Object Oriented Programming.mp4
00:00 -
004 Factory Functions.mp4
00:00 -
005 Constructor Functions.mp4
00:00 -
006 JavaScript Classes.mp4
00:00 -
007 More Classes Practice.mp4
00:00 -
008 Extends and Super Keywords.mp4
00:00 -
Section Quiz
30 – Mastering The Terminal
-
001 What Matters In This Section.mp4
00:00 -
002 Backend Overview.mp4
00:00 -
003 A Pep Talk On Terminal.mp4
00:00 -
004 Why Do We Need To Know Terminal Commands.mp4
00:00 -
005 Windows Terminal Installation Instructions.html
00:00 -
006 The Basics LS & PWD.mp4
00:00 -
007 Changing Directories.mp4
00:00 -
008 Relative Vs. Absolute Paths.mp4
00:00 -
009 Making Directories.mp4
00:00 -
010 Man Pages & Flags.mp4
00:00 -
011 The Touch Command.mp4
00:00 -
012 Removing Files & Folders.mp4
00:00 -
external-links.txt
00:00 -
Section Quiz
31 – Our First Brush With Node
-
001 What Matters In This Section.mp4
00:00 -
002 Introducing Node JS.mp4
00:00 -
003 What Is Node Used For.mp4
00:00 -
004 Installing Node.mp4
00:00 -
005 The Node REPL.mp4
00:00 -
006 Running Node Files.mp4
00:00 -
007 Process & Argv.mp4
00:00 -
008 IMPORTANT NOTE fs.writeFileSync Changes.html
00:00 -
009 File System Module Crash Course.mp4
00:00 -
external-links.txt
00:00 -
Section Quiz
32 – Exploring Modules & The NPM Universe
-
001 What Matters In This Section.mp4
00:00 -
002 Working With module.exports.mp4
00:00 -
003 Requiring A Directory.mp4
00:00 -
004 Introducing NPM.mp4
00:00 -
005 Installing Packages – Jokes & Rainbow.mp4
00:00 -
006 Adding Global Packages.mp4
00:00 -
007 The All-Important Package.json.mp4
00:00 -
008 Installing All Dependencies For A Project.mp4
00:00 -
009 IMPORTANT NOTE Fixing Issues — Language Guesser Challenge.html
00:00 -
010 Language Guesser Challenge.mp4
00:00 -
external-links.txt
00:00 -
Section Quiz
33 – Creating Servers With Express
-
001 What Matters In This Section.mp4
00:00 -
002 Introducing Express.mp4
00:00 -
003 Our Very First Express App.mp4
00:00 -
004 The Request & Response Objects.mp4
00:00 -
005 Express Routing Basics.mp4
00:00 -
006 Express Path Parameters.mp4
00:00 -
007 Working With Query Strings.mp4
00:00 -
008 Auto-Restart With Nodemon.mp4
00:00 -
external-links.txt
00:00 -
Section Quiz
34 – Creating Dynamic HTML With Templating
-
001 What Matters In This Section.mp4
00:00 -
002 What is Templating.mp4
00:00 -
003 Configuring Express For EJS.mp4
00:00 -
004 Setting The Views Directory.mp4
00:00 -
005 EJS Interpolation Syntax.mp4
00:00 -
006 Passing Data To Templates.mp4
00:00 -
007 Subreddit Template Demo.mp4
00:00 -
008 Conditionals in EJS.mp4
00:00 -
009 Loops In EJS.mp4
00:00 -
010 A More Complex Subreddit Demo.mp4
00:00 -
011 Serving Static Assets In Express.mp4
00:00 -
012 Bootstrap + Express.mp4
00:00 -
013 EJS & Partials.mp4
00:00 -
external-links.txt
00:00 -
Section Quiz
35 – Defining RESTful Routes
-
001 What Matters In This Section.mp4
00:00 -
002 Get Vs. Post Requests.mp4
00:00 -
003 Defining Express Post Routes.mp4
00:00 -
004 Parsing The Request Body.mp4
00:00 -
005 Intro to REST.mp4
00:00 -
006 RESTful Comments Overview.mp4
00:00 -
007 RESTful Comments Index.mp4
00:00 -
008 RESTful Comments New.mp4
00:00 -
009 Express Redirects.mp4
00:00 -
010 RESTful Comments Show.mp4
00:00 -
011 The UUID Package.mp4
00:00 -
012 RESTful Comments Update.mp4
00:00 -
013 Express Method Override.mp4
00:00 -
014 RESTful Comments Delete.mp4
00:00 -
external-links.txt
00:00 -
Section Quiz
36 – Our First Database MongoDB
-
001 What Matters In This Section.mp4
00:00 -
002 Introduction to Databases.mp4
00:00 -
003 SQL Vs. NoSQL Databases.mp4
00:00 -
004 Why We’re Learning Mongo.mp4
00:00 -
005 Installing Mongo MacOS.mp4
00:00 -
006 Installing Mongo Windows Installation Tutorial.html
00:00 -
007 IMPORTANT NOTE About The Mongo Shell.html
00:00 -
008 The Mongo Shell.mp4
00:00 -
009 What On Earth Is BSON.mp4
00:00 -
010 Inserting With Mongo.mp4
00:00 -
011 Finding With Mongo.mp4
00:00 -
012 Updating With Mongo.mp4
00:00 -
013 Deleting With Mongo.mp4
00:00 -
014 Additional Mongo Operators.mp4
00:00 -
external-links.txt
00:00 -
Section Quiz
37 – Connecting To Mongo With Mongoose
-
001 What Matters In This Section.mp4
00:00 -
002 What is Mongoose.mp4
00:00 -
003 A note about solving mongoose connection issues.html
00:00 -
004 Connecting Mongoose to Mongo.mp4
00:00 -
005 A note about the node .load index.js command issue.html
00:00 -
006 Our First Mongoose Model.mp4
00:00 -
007 Insert Many.mp4
00:00 -
008 Finding With Mongoose.mp4
00:00 -
009 Updating With Mongoose.mp4
00:00 -
010 Deleting With Mongoose!.mp4
00:00 -
011 Mongoose Schema Validations.mp4
00:00 -
012 Additional Schema Constraints.mp4
00:00 -
013 Validating Mongoose Updates.mp4
00:00 -
014 Mongoose Validation Errors.mp4
00:00 -
015 Model Instance Methods.mp4
00:00 -
016 Adding Model Static Methods.mp4
00:00 -
017 Mongoose Virtuals.mp4
00:00 -
018 Defining Mongoose Middleware.mp4
00:00 -
external-links.txt
00:00 -
Section Quiz
38 – Putting It All Together Mongoose With Express
-
001 What Matters In This Section.mp4
00:00 -
002 Express + Mongoose Basic Setup.mp4
00:00 -
003 Creating Our Model.mp4
00:00 -
004 Products Index.mp4
00:00 -
005 Product Details.mp4
00:00 -
006 Creating Products.mp4
00:00 -
007 Updating Products.mp4
00:00 -
008 Tangent On Category Selector.mp4
00:00 -
009 Deleting Products.mp4
00:00 -
010 BONUS Filtering By Category.mp4
00:00 -
Section Quiz
39 – YelpCamp Campgrounds CRUD
-
001 Introducing YelpCamp Our Massive Project.mp4
00:00 -
002 How to Access YelpCamp Code.mp4
00:00 -
003 Creating the Basic Express App.mp4
00:00 -
004 Campground Model Basics.mp4
00:00 -
005 Seeding Campgrounds.mp4
00:00 -
006 Campground Index.mp4
00:00 -
007 Campground Show.mp4
00:00 -
008 Campground New & Create.mp4
00:00 -
009 Campground Edit & Update.mp4
00:00 -
010 Campground Delete.mp4
00:00 -
external-links.txt
00:00 -
Section Quiz
40 – Middleware The Key To Express
-
001 What Matters In This Section.mp4
00:00 -
002 Intro to Express Middleware.mp4
00:00 -
003 Using Morgan – Logger Middleware.mp4
00:00 -
004 Defining Our Own Middleware.mp4
00:00 -
005 More Middleware Practice.mp4
00:00 -
006 Setting Up A 404 Route.mp4
00:00 -
007 Password Middleware Demo (NOT REAL AUTH).mp4
00:00 -
008 Protecting Specific Routes.mp4
00:00 -
external-links.txt
00:00 -
Section Quiz
41 – YelpCamp Adding Basic Styles
-
001 A New EJS Tool For Layouts.mp4
00:00 -
002 Bootstrap5! Boilerplate.mp4
00:00 -
003 Navbar Partial.mp4
00:00 -
004 Footer Partial.mp4
00:00 -
005 Adding Images.mp4
00:00 -
006 Styling Campgrounds Index.mp4
00:00 -
007 Styling The New Form.mp4
00:00 -
008 Styling Edit Form.mp4
00:00 -
009 Styling Show Page.mp4
00:00 -
external-links.txt
00:00 -
Section Quiz
42 – Handling Errors In Express Apps
-
001 What Matters In This Section.mp4
00:00 -
002 Express’ Built-In Error Handler.mp4
00:00 -
003 Defining Custom Error Handlers.mp4
00:00 -
004 Our Custom Error Class.mp4
00:00 -
005 Handling Async Errors.mp4
00:00 -
006 Handling More Async Errors!.mp4
00:00 -
007 Defining An Async Utility.mp4
00:00 -
008 Differentiating Mongoose Errors.mp4
00:00 -
Section Quiz
43 – YelpCamp Errors & Validating Data
-
001 Where To Next With YelpCamp.mp4
00:00 -
002 Client-Side Form Validations.mp4
00:00 -
003 Basic Error Handler.mp4
00:00 -
004 Defining ExpressError Class.mp4
00:00 -
005 More Errors.mp4
00:00 -
006 Defining Error Template.mp4
00:00 -
007 JOI Schema Validations.mp4
00:00 -
008 JOI Validation Middleware.mp4
00:00 -
external-links.txt
00:00 -
Section Quiz
44 – Data Relationships With Mongo
-
001 What Matters In This Section.mp4
00:00 -
002 Introduction to Mongo Relationships.mp4
00:00 -
003 SQL Relationships Overview.mp4
00:00 -
004 Note Solving an upcoming code issue with mongoose version 7.html
00:00 -
005 One to Few.mp4
00:00 -
006 One to Many.mp4
00:00 -
007 Mongoose Populate.mp4
00:00 -
008 One to Bajillions.mp4
00:00 -
009 Mongo Schema Design.mp4
00:00 -
external-links.txt
00:00 -
Section Quiz
45 – Mongo Relationships With Express
-
001 What Matters In This Section.mp4
00:00 -
002 Defining Our Farm & Product Models.mp4
00:00 -
003 Note about Farm model.html
00:00 -
004 Creating New Farms.mp4
00:00 -
005 Farms Show Page.mp4
00:00 -
006 Creating Products For A Farm.mp4
00:00 -
007 Finishing Touches.mp4
00:00 -
008 Deletion Mongoose Middleware.mp4
00:00 -
Section Quiz
46 – YelpCamp Adding The Reviews Model
-
001 Defining The Review Model.mp4
00:00 -
002 Adding The Review Form.mp4
00:00 -
003 Creating Reviews.mp4
00:00 -
004 Validating Reviews.mp4
00:00 -
005 Displaying Reviews.mp4
00:00 -
006 Styling Reviews.mp4
00:00 -
007 Deleting Reviews.mp4
00:00 -
008 Campground Delete Middleware.mp4
00:00 -
external-links.txt
00:00 -
Section Quiz
47 – Express Router & Cookies
-
001 What Matters In This Section.mp4
00:00 -
002 Express Router Intro.mp4
00:00 -
003 Express Router & Middleware.mp4
00:00 -
004 Introducing Cookies.mp4
00:00 -
005 Sending Cookies.mp4
00:00 -
006 Cookie Parser Middleware.mp4
00:00 -
007 Signing Cookies.mp4
00:00 -
008 OPTIONAL HMAC Signing.mp4
00:00 -
external-links.txt
00:00 -
Section Quiz
48 – Express Session & Flash
-
001 What Matters In This Section.mp4
00:00 -
002 Introduction to Sessions.mp4
00:00 -
003 Express Session.mp4
00:00 -
004 More Express Session.mp4
00:00 -
005 Intro to Flash.mp4
00:00 -
006 Res.locals & Flash.mp4
00:00 -
external-links.txt
00:00 -
Section Quiz
49 – YelpCamp Restructuring & Flash
-
001 Breaking Out Campground Routes.mp4
00:00 -
002 Breaking Out Review Routes.mp4
00:00 -
003 Serving Static Assets.mp4
00:00 -
004 Configuring Session.mp4
00:00 -
005 Setting Up Flash.mp4
00:00 -
006 Flash Success Partial.mp4
00:00 -
007 Flash Errors Partial.mp4
00:00 -
external-links.txt
00:00 -
Section Quiz
50 – Authentication From Scratch
-
001 What Matters In This Section.mp4
00:00 -
002 Authentication Vs. Authorization.mp4
00:00 -
003 How to (not) Store Passwords.mp4
00:00 -
004 Cryptographic Hashing Functions.mp4
00:00 -
005 Password Salts.mp4
00:00 -
006 Intro to Bcrypt.mp4
00:00 -
007 Auth Demo Setup.mp4
00:00 -
008 Auth Demo Registering.mp4
00:00 -
009 Auth Demo Login.mp4
00:00 -
010 Auth Demo Staying Logged In With Session.mp4
00:00 -
011 Auth Demo Logout.mp4
00:00 -
012 Auth Demo Require Login Middleware.mp4
00:00 -
013 Auth Demo Refactoring To Model Methods.mp4
00:00 -
external-links.txt
00:00 -
Section Quiz
51 – YelpCamp Adding In Authentication
-
001 Introduction to Passport.mp4
00:00 -
002 Creating Our User Model.mp4
00:00 -
003 Configuring Passport.mp4
00:00 -
004 Register Form.mp4
00:00 -
005 Register Route Logic.mp4
00:00 -
006 Login Routes.mp4
00:00 -
007 isLoggedIn Middleware.mp4
00:00 -
008 IMPORTANT Fixing Logout.html
00:00 -
009 Adding Logout.mp4
00:00 -
010 currentUser Helper.mp4
00:00 -
011 Fixing Register Route.mp4
00:00 -
012 IMPORTANT Passport.js Updates — Fixing The returnToRedirect Issue.html
00:00 -
013 ReturnTo Behavior.mp4
00:00 -
external-links.txt
00:00 -
Section Quiz
52 – YelpCamp Basic Authorization
-
001 Adding an Author to Campground.mp4
00:00 -
002 Showing and Hiding EditDelete.mp4
00:00 -
003 Campground Permissions.mp4
00:00 -
004 Authorization Middleware.mp4
00:00 -
005 Reviews Permissions.mp4
00:00 -
006 More Reviews Authorization.mp4
00:00 -
external-links.txt
00:00 -
Section Quiz
53 – YelpCamp Controllers & Star Ratings
-
001 Refactoring To Campgrounds Controller.mp4
00:00 -
002 Adding a Reviews Controller.mp4
00:00 -
003 A Fancy Way To Restructure Routes.mp4
00:00 -
004 Displaying Star Ratings.mp4
00:00 -
005 Star Rating Form.mp4
00:00 -
external-links.txt
00:00 -
Section Quiz
54 – YelpCamp Image Upload
-
001 Intro To Image Upload Process.mp4
00:00 -
002 The Multer Middleware.mp4
00:00 -
003 Cloudinary Registration.mp4
00:00 -
004 Environment Variables with dotenv.mp4
00:00 -
005 IMPORTANT NOTE Fixing npm install Issues In The Next Lecture.html
00:00 -
006 Uploading To Cloudinary Basics.mp4
00:00 -
007 Storing Uploaded Image Links In Mongo.mp4
00:00 -
008 Displaying Images In A Carousel.mp4
00:00 -
009 Fixing Our Seeds.mp4
00:00 -
010 Adding Upload to Edit Page.mp4
00:00 -
011 Customizing File Input.mp4
00:00 -
012 A Word Of Warning!.mp4
00:00 -
013 Deleting Images Form.mp4
00:00 -
014 Deleting Images Backend.mp4
00:00 -
015 Adding a Thumbnail Virtual Property.mp4
00:00 -
external-links.txt
00:00 -
Section Quiz
55 – YelpCamp Adding Maps
-
001 Registering For Mapbox.mp4
00:00 -
002 Geocoding Our Locations.mp4
00:00 -
003 Working With GeoJSON.mp4
00:00 -
004 Displaying A Map.mp4
00:00 -
005 Centering The Map On A Campground.mp4
00:00 -
006 Fixing Our Seeds Bug.mp4
00:00 -
007 Customizing Map Popup.mp4
00:00 -
external-links.txt
00:00 -
Section Quiz
56 – YelpCamp Fancy Cluster Map
-
001 Intro To Our Cluster Map.mp4
00:00 -
002 Adding Earthquake Cluster Map.mp4
00:00 -
003 Reseeding Our Database (again).mp4
00:00 -
004 Basic Clustering Campgrounds.mp4
00:00 -
005 Tweaking Clustering Code.mp4
00:00 -
006 Changing Cluster Size and Color.mp4
00:00 -
007 Adding Custom Popups.mp4
00:00 -
external-links.txt
00:00 -
Section Quiz
57 – YelpCamp Styles Clean Up
-
001 Styling Home Page.mp4
00:00 -
002 Additional Home Page Styling.mp4
00:00 -
003 Styling Login Form.mp4
00:00 -
004 Styling Register Form.mp4
00:00 -
005 Spacing Campgrounds.mp4
00:00 -
006 Removing Inline Map Styles.mp4
00:00 -
007 Adding Map Controls.mp4
00:00 -
external-links.txt
00:00 -
Section Quiz
58 – YelpCamp Common Security Issues
-
001 Mongo Injection.mp4
00:00 -
002 Cross Site Scripting (XSS).mp4
00:00 -
003 Sanitizing HTML w JOI.mp4
00:00 -
004 Minor Changes to SessionCookies.mp4
00:00 -
005 Hiding Errors.mp4
00:00 -
006 IMPORTANT Helmet Updates — Fixing Issues.html
00:00 -
007 Using Helmet.mp4
00:00 -
008 Content Security Policy Fun.mp4
00:00 -
external-links.txt
00:00 -
Section Quiz
59 – YelpCamp Deploying
-
001 Setting Up Mongo Atlas.mp4
00:00 -
002 IMPORTANT connect-mongo — Handling Updates.html
00:00 -
003 Using Mongo For Our Session Store.mp4
00:00 -
004 Render — A free alternative for deploying your app.html
00:00 -
005 Heroku Setup.mp4
00:00 -
006 Pushing to Heroku.mp4
00:00 -
007 Fixing Heroku Errors.mp4
00:00 -
008 Configuring Heroku Env Variables.mp4
00:00 -
external-links.txt
00:00 -
Section Quiz
60 – Introducing React
-
001 How The React Content Works.mp4
00:00 -
002 React Content Slides.html
00:00 -
003 Introducing React.mp4
00:00 -
004 Setting Up Code Sand Box.mp4
00:00 -
005 The Basics of JSX.mp4
00:00 -
006 Basic React App Structure.mp4
00:00 -
007 Our First React Component.mp4
00:00 -
external-links.txt
00:00 -
Section Quiz
61 – JSX In Detail
-
001 Importing and Exporting Components.mp4
00:00 -
002 The Rules of JSX.mp4
00:00 -
003 React Fragments.mp4
00:00 -
004 Evaluating JS Expressions in JSX.mp4
00:00 -
005 Creating. a Die Component.mp4
00:00 -
006 Component Decomposition.mp4
00:00 -
007 Styling Components.mp4
00:00 -
008 Random PokeCard Exercise.mp4
00:00 -
009 GET THE POKEMON IMAGE URL HERE.html
00:00 -
external-links.txt
00:00 -
Section Quiz
62 – Local React Apps With Vite
-
001 Creating React Apps With Vite.mp4
00:00 -
002 A Note About Create React App.mp4
00:00 -
003 A Tour of A Vite App.mp4
00:00 -
external-links.txt
00:00 -
Section Quiz
63 – Working With Props
-
001 Introducing Props.mp4
00:00 -
002 Non-String Props.mp4
00:00 -
003 Setting Default Prop Values.mp4
00:00 -
004 Passing Arrays and Objects.mp4
00:00 -
005 React Conditionals.mp4
00:00 -
006 The React Developer Tools.mp4
00:00 -
007 Adding Dynamic Component Styles.mp4
00:00 -
008 Rendering Arrays With Map.mp4
00:00 -
009 Slot Machine Exercise.mp4
00:00 -
Section Quiz
64 – Shopping List Demo keys, prop types, and more!
-
001 Creating A Shopping List Component.mp4
00:00 -
002 The Key Prop.mp4
00:00 -
003 The Shopping List Item Component.mp4
00:00 -
004 Rental Property Exercise.mp4
00:00 -
005 Rental Properties Exercise STARTER DATA.html
00:00 -
006 Configuring ESLint.mp4
00:00 -
007 PropTypes Library Crash Course.mp4
00:00 -
Section Quiz
65 – React Events
-
001 Intro to React Events.mp4
00:00 -
002 Non-Click Events.mp4
00:00 -
003 Working With The Event Object.mp4
00:00 -
004 Clicker Exercise.mp4
00:00 -
Section Quiz
66 – The Basics of React State
-
001 Introducing State.mp4
00:00 -
002 Working With The useState() Hook.mp4
00:00 -
003 Creating a Toggler Component.mp4
00:00 -
004 Multiple Pieces of State In A Component.mp4
00:00 -
005 useState() and Rendering.mp4
00:00 -
006 Color Box Exercise INTRO.mp4
00:00 -
007 Color Box Exercise SOLUTION.mp4
00:00 -
Section Quiz
67 – Intermediate State Concepts
-
001 Setting State With An Updater Function.mp4
00:00 -
002 State Initializer Functions.mp4
00:00 -
003 When Does React Re-Render.mp4
00:00 -
004 Working With Objects In State.mp4
00:00 -
005 Arrays In State.mp4
00:00 -
006 Generating Ids with UUID.mp4
00:00 -
007 Deleting From Arrays The React Way.mp4
00:00 -
008 Common Array Updating Patterns.mp4
00:00 -
009 Updating All Elements In An Array.mp4
00:00 -
010 Score Keeper Exercise.mp4
00:00 -
Section Quiz
68 – Component Design
-
001 Introducing The Lucky7 Game.mp4
00:00 -
002 Formulating Our Component Gameplan.mp4
00:00 -
003 Building Die and Dice Components.mp4
00:00 -
004 The LuckyN Component.mp4
00:00 -
005 State As Props.mp4
00:00 -
006 Passing Functions As Props.mp4
00:00 -
007 Passing Functions That Update State.mp4
00:00 -
008 Practice Passing State Updating Functions.mp4
00:00 -
Section Quiz
69 – React Forms
-
001 Controlled Components.mp4
00:00 -
002 The htmlFor Property.mp4
00:00 -
003 Working With Multiple Inputs.mp4
00:00 -
004 A Better Signup Form.mp4
00:00 -
005 Computed Property Names in HandleChange().mp4
00:00 -
006 Creating a Shopping List Form.mp4
00:00 -
007 Shopping List Component.mp4
00:00 -
008 Finishing The Shopping List Component.mp4
00:00 -
009 Form Validation From Scratch.mp4
00:00 -
010 Validations using React Hook Form.mp4
00:00 -
Section Quiz
70 – Effects
-
001 Introducing Effects.mp4
00:00 -
002 The useEffect Hook.mp4
00:00 -
003 useEffect Dependencies.mp4
00:00 -
004 Fetching Initial Data From an API.mp4
00:00 -
005 Adding a Loader.mp4
00:00 -
006 Github Profile Search.mp4
00:00 -
Section Quiz
71 – Material UI
-
001 Introducing Material UI.mp4
00:00 -
002 Installing Material UI.mp4
00:00 -
003 The Rating Component.mp4
00:00 -
004 Material Forms.mp4
00:00 -
005 The SX Prop and Custom Styles.mp4
00:00 -
Section Quiz
72 – Building a Todo List With Material UI & Local Storage
-
001 Creating The Application.mp4
00:00 -
002 The TodoList Component.mp4
00:00 -
003 Removing Todos.mp4
00:00 -
004 Toggling Todos.mp4
00:00 -
005 The New Todo Form.mp4
00:00 -
006 Adding LocalStorage.mp4
00:00 -
007 Tweaking the Todo Icons and Ids.mp4
00:00 -
008 Final Styles.mp4
00:00 -
Section Quiz
73 – BONUS Fancy, More Advanced Todolist
-
001 Check Out This Fancy Todo List.mp4
00:00
74 – The End (
-
001 What’s Next and Goodbye!.mp4
00:00
Earn a certificate
Add this certificate to your resume to demonstrate your skills & increase your chances of getting noticed.
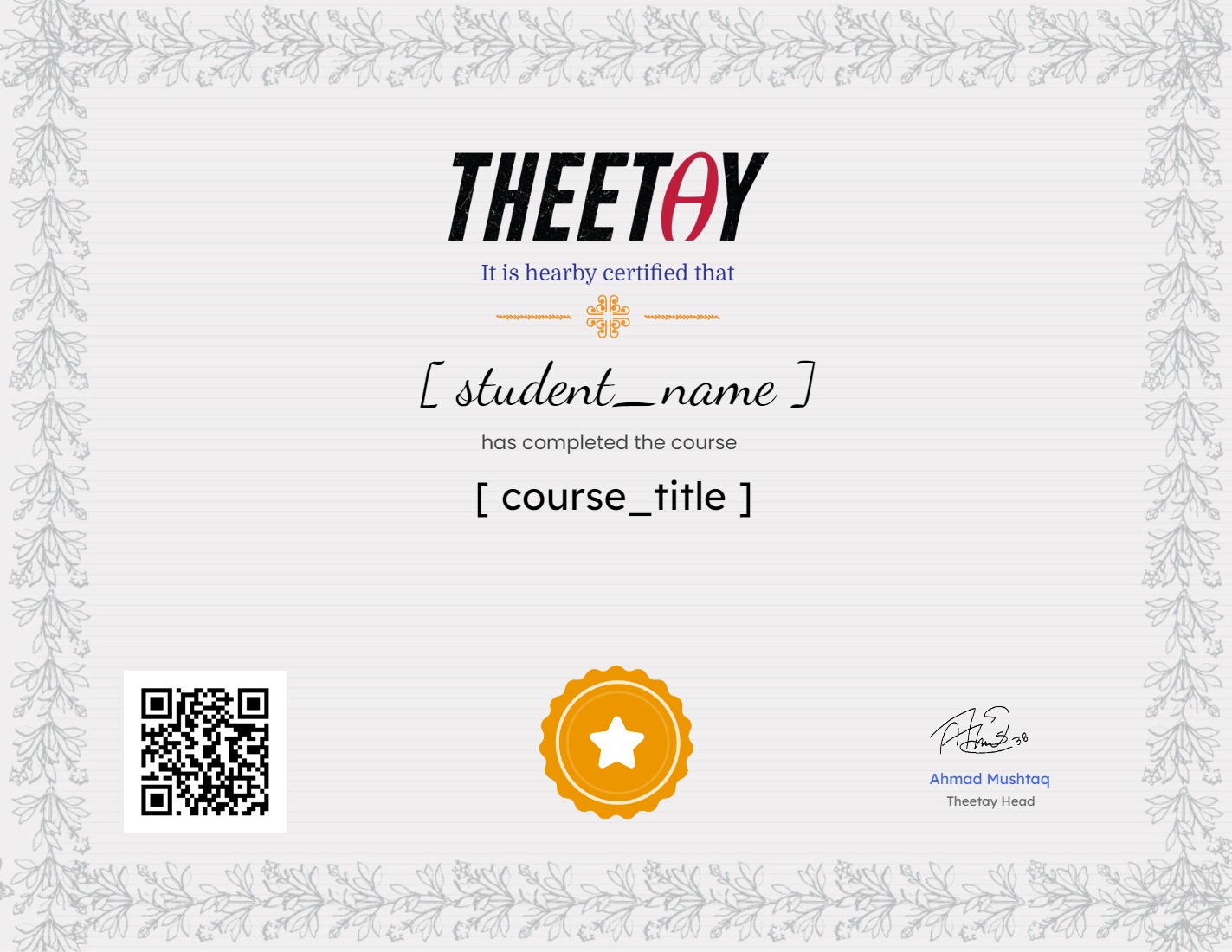